example : 1 code using System;
// compare a structure. and clastrcuts
//compare_struct_class_2.cs
struct MyStruct {
public int x;
}
class MyClass {
public int x;
}
// Demonstrate structure astrcutsignment.
class compare {
public static void Main() {
MyStruct astrcut = new MyStruct();
MyStruct bstrcut = new MyStruct();
astrcut.x = 10;
bstrcut.x = 20;
Console.WriteLine("--struct out put--");
Console.WriteLine("astrcut.x {0}, bstrcut.x {1}", astrcut.x, bstrcut.x);
astrcut = bstrcut;
bstrcut.x = 30;
Console.WriteLine("astrcut.x {0}, bstrcut.x {1}", astrcut.x, bstrcut.x);
MyClass aclass = new MyClass();
MyClass bclass = new MyClass();
aclass.x = 10;
bclass.x = 20;
Console.WriteLine("--class out put--");
Console.WriteLine("aclass.x {0}, bclass.x {1}", aclass.x, bclass.x);
aclass = bclass;
bclass.x = 30;
Console.WriteLine("aclass.x {0}, bclass.x {1}", aclass.x, bclass.x);
}
}
|
Note the data copies only value with struct, on the other hand class
changes at the level of reference point of the variable "aclass";
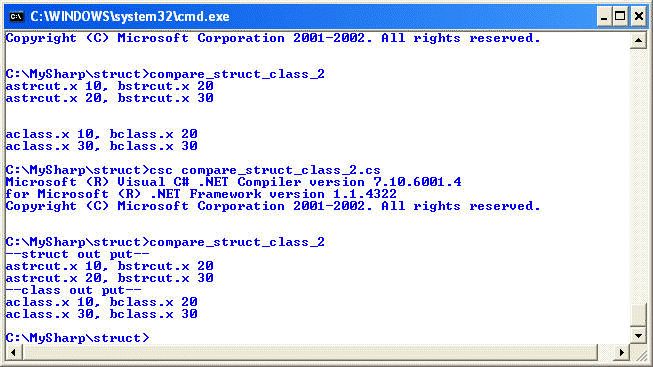
Passing by reference means (
from right to left ), variable from the right was
pointing to stored memory
location of a variable on the left, any change at the memory location
will replace original/previous value
with the current one. However, during
passing by value, the variable from the right side of equation sign,
does not access the memory
location of the target object, therefore old values in the variable on left
side will not change.
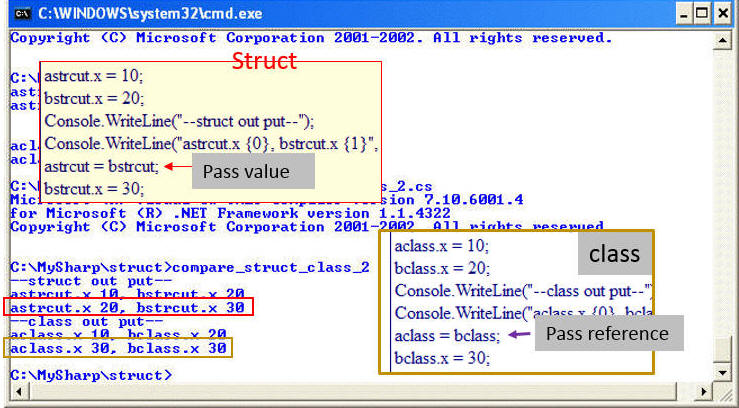 |
This example I found from the internet and please call, I would like to
refer the contributor or delete if it violates any law whatsoever. using
System;
//compare_struct_class.cs
//this is taken from MSDN sties
//http://msdn2.microsoft.com/en-us/library/8b0bdca4.aspx
class TheClass
{
public string willIChange;
}
struct TheStruct
{
public string willIChange;
}
class TestClassAndStruct
{
static void ClassTaker(TheClass c)
{
c.willIChange = "Changed";
}
static void StructTaker(TheStruct s)
{
s.willIChange = "Changed";
}
static void Main()
{
TheClass testClass = new TheClass();
TheStruct testStruct = new TheStruct();
testClass.willIChange = "Not Changed";
testStruct.willIChange = "Not Changed";
ClassTaker(testClass);
StructTaker(testStruct);
System.Console.WriteLine("Class field = {0}", testClass.willIChange);
System.Console.WriteLine("Struct field = {0}", testStruct.willIChange);
}
} |