csharp_struct_1.htm Next |
Why structures !!
Compared with Class
- Struct is less capable of version of a class, but more efficient in
performance wise comparing with its class version.
- Struct is a value type and
Class is Reference type, therefore Struct operates directly, without
suffering any performance loss. . The image below shows the resemblances

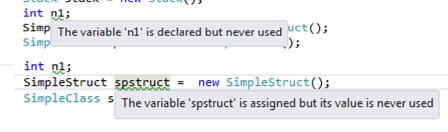
- The New operator is used to instantiate class and stack. The
instance in STRUCT object is stored in
Stack, but New
Operator with Class, the object is allocated in
HEAP
- Struct does not create overhead and if you
don’t have use inheritance and need to store a small group of data, struct
is a better choice over class.
|
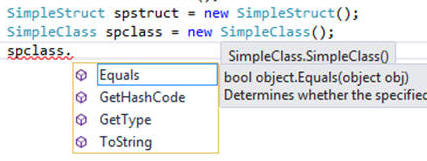 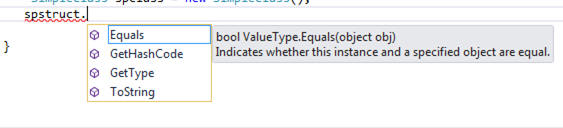
|
|
Struct: Structs are a type of composite data structures composed of several pieces of data, possibly
different types.
Defining structs:
Struct <typename>
{
//member Declarations
} |
Some Info on struct:
- Strcts are value types and classes are reference type
- A struct object, goes to stack, and it is not allocated on the managed heap, and therefore
it is not subject to garbage collection. When we create a new struct object, the
new operator is NOT actually invoked. The matrix directly allocated to the
function. It is created when a function begins execution,
and ceases to exist when function terminates.
- Every value type is automatically provided with a default constructor
that is a constructor that takes no argument. The default constructor
zeros out the data members stored with the object of the value type. It is
an error to provide an explicit default constructor within a struct
definition.
- Invoking the new operator on a value type simply results in the
execution of an associated constructor. If no arguments are passed to the
invocation, the default constructor is invoked. If we provide arguments
the compiler searches for a constructor matching those arguments.
|
Struct : |
using System;
//struct_simple.cs
//my example on the fly.
namespace simplestruc
{
struct empdb
{
public int id;
public string name;
public int age;
public float sal;
public empdb(int eid,string ename, int eage, float esal){
this.id = eid;
this.name = ename;
this.age = eage;
this.sal = esal;
}
}
class testStruc {
public static void Main()
{
empdb empinfo = new empdb();
empinfo.age = 45;
empinfo.name = "Manas";
empinfo.id = 237;
empinfo.sal = 4500;
//
Console.WriteLine("\n Employe ID : " + empinfo.id);
Console.WriteLine("\n Employe ID : " + empinfo.name);
Console.WriteLine("\n Employe age : " + empinfo.age);
} } }
|
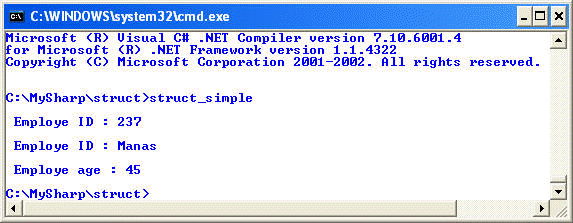 |
Simple structure with user's input |
code using System;
//struct_simple_read.cs
//my example on the fly.
namespace simplestruc
{
struct playerdb
{
public int id;
public string name;
public int age;
public float sal;
public playerdb(int eid,string ename, int eage, float esal){
this.id = eid;
this.name = ename;
this.age = eage;
this.sal = esal;
}
}
class testStruc {
public static void Main()
{
playerdb playerinfo = new playerdb();
playerinfo.age = 45;
playerinfo.name = "Manas";
playerinfo.id = 237;
playerinfo.sal = 4500;
//
Console.WriteLine("player ID : " + playerinfo.id);
Console.WriteLine("player name : " + playerinfo.name);
Console.WriteLine("player age : " + playerinfo.age);
Console.WriteLine("player sal : " + playerinfo.sal);
//
Console.Write("\n Do you want to add a new player(0=No/1=Yes)? ");
int answer = int.Parse(Console.ReadLine());
//if text there will be an error
if( answer == 1 ){
Console.Write("enter players id : "); playerinfo.id =
int.Parse(Console.ReadLine());
Console.Write("enter players name : "); playerinfo.name=Console.ReadLine();
Console.Write("enter players age : "); playerinfo.age=int.Parse(Console.ReadLine());
Console.Write("enter players sal : "); playerinfo.sal=float.Parse(Console.ReadLine());
}
//
Console.WriteLine("\n ---New Player added------ ");
Console.WriteLine("player ID : " + playerinfo.id);
Console.WriteLine("player Name : " + playerinfo.name);
Console.WriteLine("player age : " + playerinfo.age);
Console.WriteLine("player sal : " + playerinfo.sal);
//
} } }
|
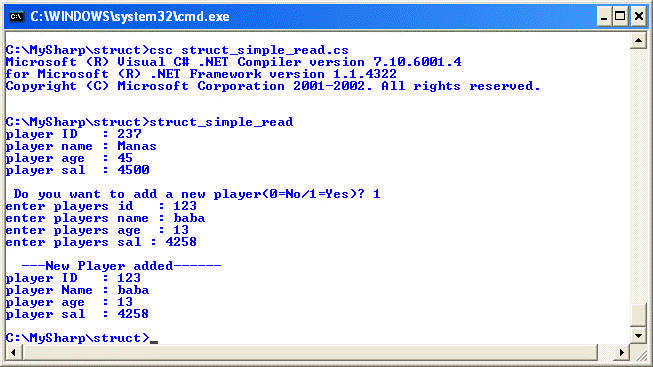 |
|
|
|
|
|
|