Prev |
csharp_struct_3.htm |
using System;
//also calling method
//csc struct_constructor.cs
namespace structconsructor2
{
struct MP
{
//constructor does not use return type
public int x, y;
public MP(int xx)
{
x = xx;
y = 12;
Console.WriteLine("---1st Constructor---");
}
public MP(int xx, int yy)
{
x = xx;
y = yy * x;
Console.WriteLine("----2nd Constructor---");
}
}
class cMP {
public static void Main()
{
Console. WriteLine("Nothing new about this example just to note that");
Console. WriteLine("a style of overriding struct with the same method name
MP");
int num1, num2;
Console. WriteLine("Enter a Number");
string str = Console.ReadLine();
num1 = Int32.Parse(str);
Console. WriteLine("Enter another Number");
str = Console.ReadLine();
num2 = Int32.Parse(str);
//now create an object with no argument
MP oMP1 = new MP();
Console.WriteLine(oMP1.x + "<> " + oMP1.y);
MP oMP2 = new MP(num1);
Console.WriteLine(oMP2.x + " " + oMP2.y);
MP oMP3 = new MP(num1,num2);
Console.WriteLine(oMP3.x + " " + oMP3.y);
}
}//end of class
}//end of namespace
|
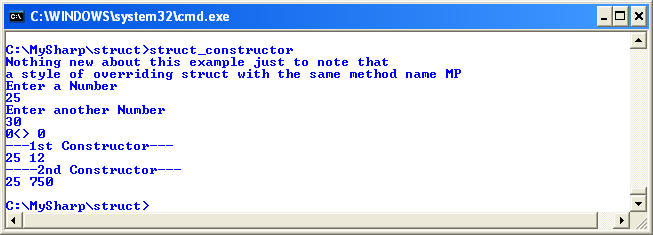 |
If you note 0<>0, is the product of
MP oMP1 = new MP();
Console.WriteLine(oMP1.x + "<> " + oMP1.y); |
|
Now use as a class ( this code will throw an error, as you don't have a
constructor with no parameter) using System;
//also calling method
//csc class_conclassor.cs
namespace classconsructor
{
class MP
{
//conclassor does not use return type
public int x, y;
public MP(int xx)
{
x = xx;
y = 12;
Console.WriteLine("---1st Conclassor---");
}
public MP(int xx, int yy)
{
x = xx;
y = yy * x;
Console.WriteLine("----2nd Conclassor---");
}
}
class cMP {
public static void Main()
{
Console. WriteLine("Nothing new about this example just to note that");
Console. WriteLine("a style of overriding class with the same method name
MP");
int num1, num2;
Console. WriteLine("Enter a Number");
string str = Console.ReadLine();
num1 = Int32.Parse(str);
Console. WriteLine("Enter another Number");
str = Console.ReadLine();
num2 = Int32.Parse(str);
//this is not correct when it is a class
MP oMP1 = new MP();
Console.WriteLine(oMP1.x + "<> " + oMP1.y);
MP oMP2 = new MP(num1);
Console.WriteLine(oMP2.x + " " + oMP2.y);
MP oMP3 = new MP(num1,num2);
Console.WriteLine(oMP3.x + " " + oMP3.y);
}
}//end of class
}//end of namespace |
it hits the call where
MP oMP1 = new MP();
Console.WriteLine(oMP1.x + "<> " + oMP1.y);
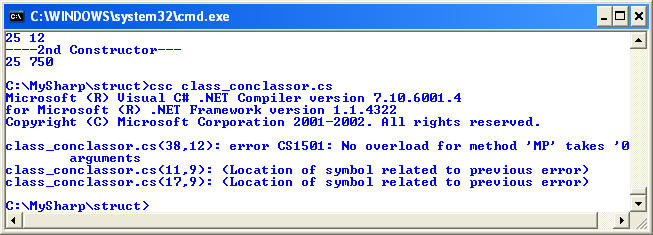
|
Now correct this code: |
using System;
//also calling method
//csc classconsructor.cs
namespace classconsructor
{
class MP
{
//conclassor does not use return type
public int x, y;
public MP()
{//no parameter
Console.WriteLine("---1st Constructor---");
}
public MP(int xx)
{
x = xx;
y = 12;
Console.WriteLine("---2nd Constructor---");
}
public MP(int xx, int yy)
{
x = xx;
y = yy * x;
Console.WriteLine("----3rd Constructor---");
}
}
class cMP {
public static void Main()
{
Console. WriteLine("Nothing new about this example just to note that");
Console. WriteLine("a style of overriding class with the same method name
MP");
int num1, num2;
Console. WriteLine("Enter a Number");
string str = Console.ReadLine();
num1 = Int32.Parse(str);
Console. WriteLine("Enter another Number");
str = Console.ReadLine();
num2 = Int32.Parse(str);
MP oMP1 = new MP();
Console.WriteLine(oMP1.x + "<> " + oMP1.y);
MP oMP2 = new MP(num1);
Console.WriteLine(oMP2.x + " " + oMP2.y);
MP oMP3 = new MP(num1,num2);
Console.WriteLine(oMP3.x + " " + oMP3.y);
}
}//end of class
}//end of namespace
|
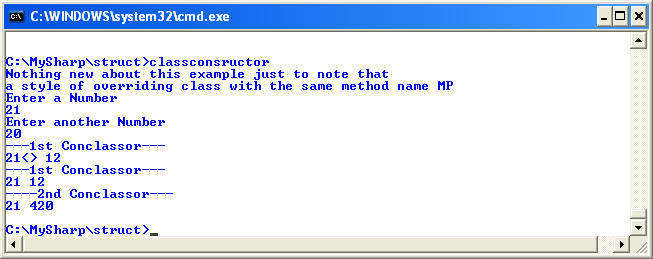 |