Overriding examples: Overriding is very loose unless derived
from an abstract class. The compiler did not complain |
|
See also
Link
|
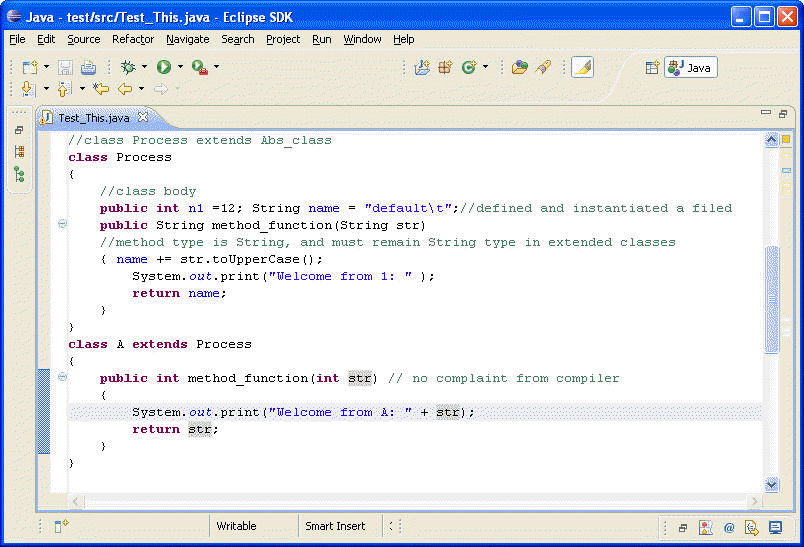 |
As I mentioned before an abstract class will complain if the
rules of overriding are not followed 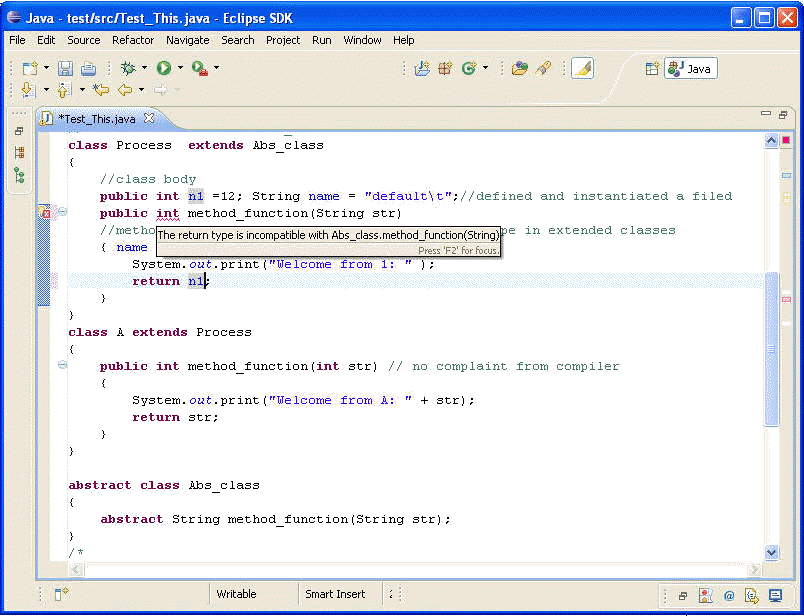
|
Further, abstraction of a method is not watched in the
derived or extended classes, can be overloaded as shown in the next
illustration. The error you see besides abstraction rules in the extended
class A, was due to wrong return type (see next illustration)
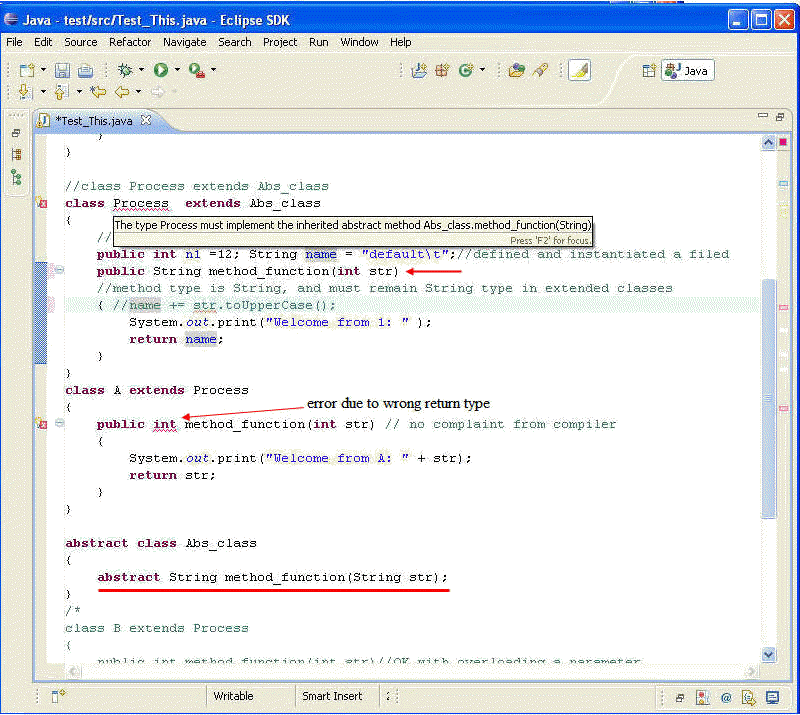
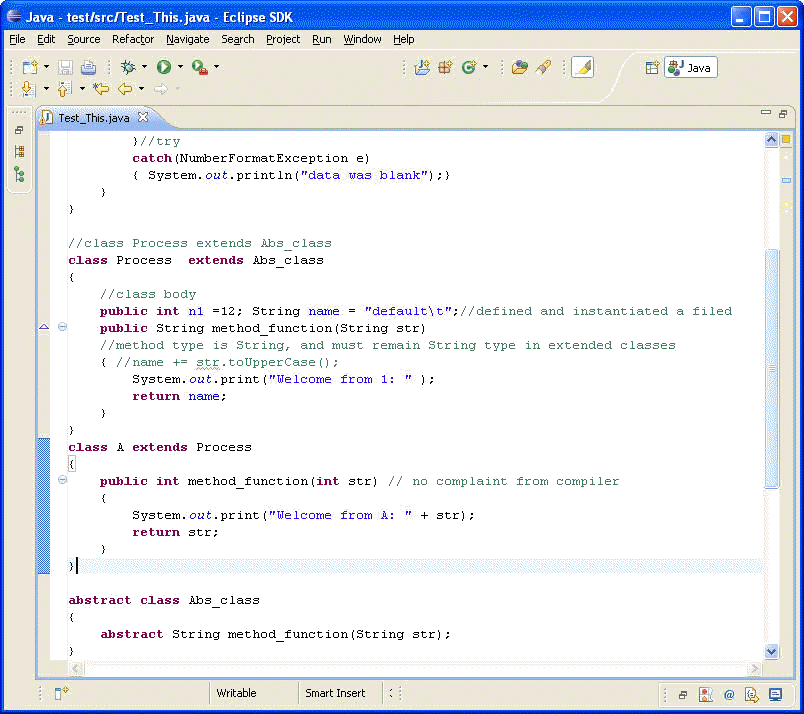
|
Together with overriding and overloading we experience a
great deal of flexibility to manipulate our routines. |
import java.io.*;
//import java.lang.reflect.*;
public class Test_This {
public static void main(String[] args) throws IOException{
// TODO Auto-generated method stub
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
String str ="", str1="";int n1=0, n2 = 1;
Process pp = new Process();//object is created
A aa = new A();
try{
System.out.print("Please write your name: ");
str = br.readLine();
System.out.println("\t"+ pp.method_function(str));
System.out.println("\t"+aa.method_function(str));
System.out.println("\t"+ aa.method_function(12));
}//try
catch(NumberFormatException e)
{ System.out.println("data was blank");}
}
}
class Process extends Abs_class
{
public int n1 =12; String name = "default\t";//defined and instantiated a
filed
public String method_function(String str)
//method type is String, and must remain String type in extended classes
{ name += str.toUpperCase();
System.out.print("Welcome from 1: " + str);
return name;
}
}
class A extends Process
{
public int method_function(int str) // no complaint from compiler
{
n1 +=str;
System.out.print("Welcome from A integer: " + str);
return n1;
}
public String method_function(String str) // no complaint from compiler
{
System.out.print("Welcome from A string: " + str);
return name;
}
}
abstract class Abs_class
{
abstract String method_function(String str);
}
|
|
|
|
|