The example below shows how an inner named class works. |
Inner named class: Link |
|
Inner anonymous class:
Link,
Link |
In the example one, a call is initiated from an
external class via an object of the class called "Process" , and
subsequently an argument in the method in local class "Process" receives
data from the caller. Now caller does not have any clue about the inner
classes or how call is being handled in the class "Process". Here call does
not go to the inner level, because it is not being used from the parent. |
Example : 1
import java.io.*;
import java.util.*;//to use EnumSet, EnumMap classes
public class Test_This {
/**
* @param args
*/
public static void main(String[] args) throws IOException{
// TODO Auto-generated method stub
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
String str ="";
Process pp = new Process();
try{
System.out.print("Please write your name: ");
str = br.readLine();
pp.a_method(str);
}//try
catch(NumberFormatException e)
{ System.out.println("data was blank");}
}
}
class Process
{
public Process() { System.out.println("Process outer constructor");}
public int n2; String name="", str_outer="";
public void a_method(String str_method)
{
str_outer = str_method;
{ System.out.println("Received call at outer method > " + str_method);
class An_inner
{
private void do_something()
{
name = this.getClass().getName();
System.out.println("Processed At inner class: " + str_outer);
}
}//end of named inner class
System.out.println("Retreive class name at inner class: " + name);
}
}//end method_1
}//end of class process
|
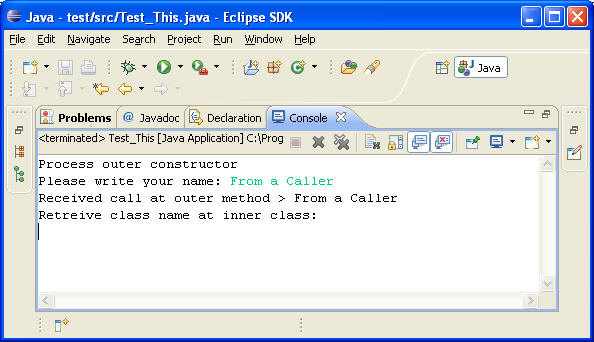 |
Example 2: |
|
import java.io.*;
import java.util.*;//to use EnumSet, EnumMap classes
public class Test_This {
/**
* @param args
*/
public static void main(String[] args) throws IOException{
// TODO Auto-generated method stub
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
String str ="";
Process pp = new Process();
try{
System.out.print("Please write your name: ");
str = br.readLine();
pp.a_method(str);
}//try
catch(NumberFormatException e)
{ System.out.println("data was blank");}
}
}
class Process
{
public Process() { System.out.println("Process outer constructor");}
public int n2; String name="", str_outer="";
public void a_method(String str_method)
{
str_outer = str_method;
System.out.println("Received call at outer method > " + str_method);
An_inner aninn = new An_inner();
aninn.do_something();
}
class An_inner
{
private void do_something()
{
name = this.getClass().getName();
System.out.println("Current class
name is: " + name);
System.out.println("Processed At inner
class: " + str_outer);
}//end of do something
}//end of An_inner class
}//end of class process
|
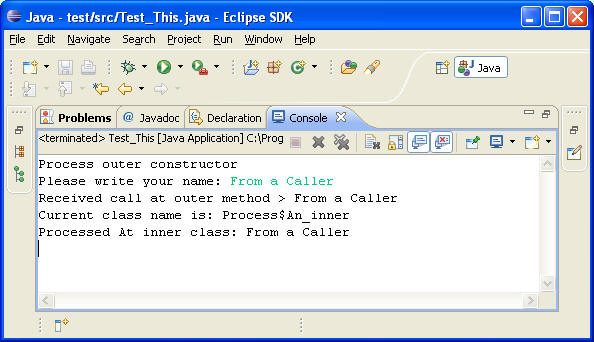 |