Thread Synchronization:threadsync_withsleep.htm |
1-2-3 |
Why needed? |
import java.util.*;
import java.io.*;
//javac test_nosync.java
public class test_nosync
{
//public static int n1 = 1;
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("-->Welcome to thread building<--");
System.out.print("type name voting zone : ");
String str = br.readLine();
System.out.println("Welcome " + str+ " to Voting Zone ");
Booth_nosync booth = new Booth_nosync();
//another way to create thread
Thread t_in = new Thread(new Voter_in_nosync(booth));
Thread t_out = new Thread(new Voter_out_nosync(booth));
t_in.start();
t_out.start();
}
}
class Booth_nosync
{
private int voter_no;
//private String voter_name;
public void voterin(int voter_in)
{
this.voter_no = voter_in;
}
public int voterout()
{
return voter_no;
}
}
class Voter_in_nosync implements Runnable
{
String name;
Booth_nosync voter;
Thread vt;
public Voter_in_nosync(Booth_nosync voter)
{
System.out.println("Voter_in_nosync Constructor");
this.voter = voter;
//System.out.println("Voter_Zone" + voter.voterzone(str));
}
public void run()
{
for(int i = 1; i < 6; i++)
{
//voter.voterin(i);
System.out.println(" Voter entered " + i);
try {
Thread.sleep(1000);
}
catch(InterruptedException e)
{
System.out.println(" lost track of voter" + e);
}
}
}
}
class Voter_out_nosync implements Runnable
{
String name;
Booth_nosync voter;
Thread vt;
public Voter_out_nosync(Booth_nosync voter)
{
System.out.println("\tVoter_out_nosync Constructor");
this.voter = voter;
}
public void run()
{
for(int i = 1; i < 6; i++)
{
//voter.voterout();
System.out.println("\t\tVoter Voted " + i);
try {
Thread.sleep(1000);
}
catch(InterruptedException e)
{
System.out.println("\t\tlost track of voter" + e);
}
}
}
}
|
The routine below, gives an imaginary situation
where voter should enter and vote in a center that allows X number of voters
at a time. In the example below, voter #3 (it may vary during another
runtime) voted before entering or registering. These events should be
synchronized, sleep provides space to let other thread work, but has some
limitations. This example also creates a scope for interacting among the
thrreads. |
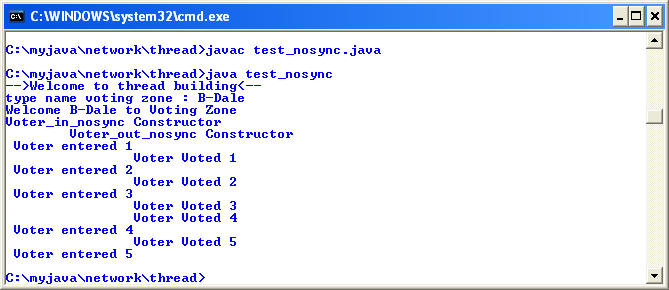 |
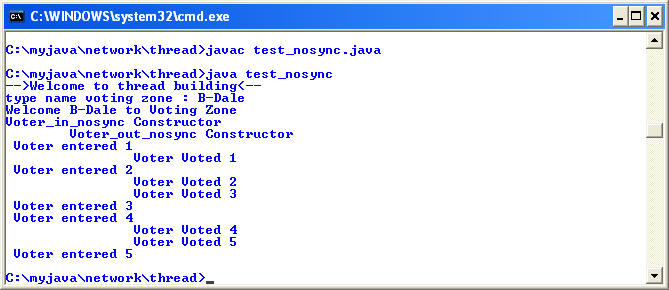 |
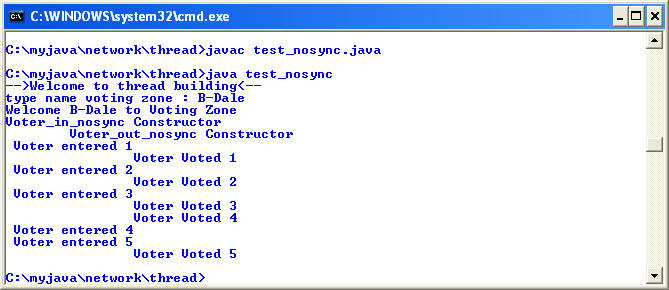 |
|
Solution: 1
Here we wanted to accomplish the followings
- All the voter in and out are taken care of, all must be all-out.
- must be registered or recognized then vote as an operation.,
using sleep 100/500 for voter_in over voter_out.
|
import java.util.*;
import java.io.*;
//javac thread_synchro.java
public class thread_synchro
{
//public static int n1 = 1;
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("-->Welcome to thread building<--");
System.out.print("type name as worker : ");
String str = br.readLine();
System.out.println("Welcome " + str+ " to Voting Zone ");
Booth_sync booth = new Booth_sync();
Thread t_in = new Thread(new Voter_in(booth));
Thread t_out = new Thread(new Voter_out_sync(booth));
t_in.start();
t_out.start();
}
}
class Booth_sync
{
private int voter_no;
public int voterin (int voter_in)
{
this.voter_no= voter_in;
return voter_no;
}
public int voterout(int voter_out)
{
this.voter_no= voter_out;
return voter_no;
}
}
class Voter_in implements Runnable
{
String name;
Booth_sync voter;
Thread vt;
public Voter_in(Booth_sync voter)
{
System.out.println("Voter_in Constructor");
this.voter = voter;
//System.out.println("Voter_Zone" + voter.voterzone(str));
}
public void run()
{
for(int i = 1; i < 10; i++)
{
try {
//voter.voterin(i);
System.out.println(" Voter registered " + voter.voterin(i));
Thread.sleep(100);
}
catch(InterruptedException e)
{
System.out.println(" lost track of voter" + e);
}
}
}
}
class Voter_out_sync implements Runnable
{
String name;
Booth_sync voter;
Thread vt;
public Voter_out_sync(Booth_sync voter)
{
System.out.println("\tVoter_out_sync Constructor");
this.voter = voter;
}
public void run()
{
for(int i = 1; i < 10; i++)
{
try {
//voter.voterout(i);
System.out.println("\t\tVoter Voted " + voter.voterout(i));
Thread.sleep(500);
}
catch(InterruptedException e)
{
System.out.println("\t\tlost track of voter" + e);
}
}
}
}
|
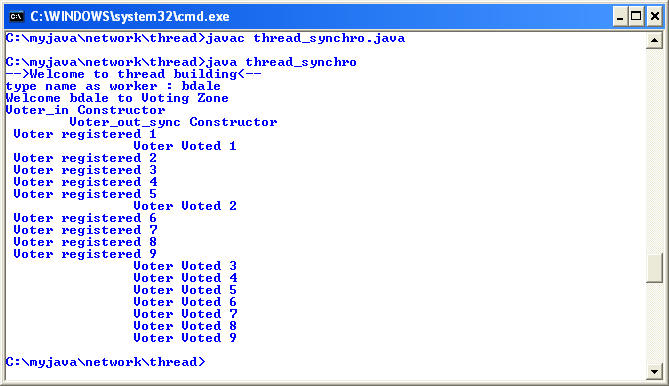 |
with Eclipse |
import java.util.*;
import java.io.*;
public class test
{
//public static int n1 = 1;
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("-->Welcome to thread building<--");
System.out.print("type name as worker : ");
String str = br.readLine();
System.out.println("Welcome " + str+ " to Voting Zone ");
Booth booth = new Booth();
Thread t_in = new Thread(new Voter_in(booth));
Thread t_out = new Thread(new Voter_out(booth));
t_in.setName(str+1);
t_out.setName(str+2);
t_in.start();
t_out.start();
}
}
class Booth
{
private int voter_no;
//private String voter_name;
//public synchronized int voterin (int voter_in)
public int voterin (int voter_in)
{
this.voter_no= voter_in;
return voter_no;
}
//public synchronized int voterout()
public int voterout(int voter_out)
{
this.voter_no= voter_out;
return voter_no;
}
}
class Voter_in implements Runnable
{
String name;
Booth voter;
Thread vt;
public Voter_in(Booth voter)
{
System.out.println("Voter_in Constructor");
this.voter = voter;
System.out.println("Thread name : " + Thread.currentThread().getName());
}
public void run()
{
int timer = (int)(Math.random()* 100);
System.out.println("Thread name void run : " +
Thread.currentThread().getName());
for(int i = 1; i < 5; i++)
{
try {
//voter.voterin(i);
System.out.println(" Voter registered " + voter.voterin(i));
System.out.println("** name : " + Thread.currentThread().getName());
Thread.sleep(timer);
}
catch(InterruptedException e)
{
System.out.println(" lost track of voter" + e);
}
}
}
}
class Voter_out implements Runnable
{
String name;
Booth voter;
Thread vt;
public Voter_out(Booth voter)
{
System.out.println("\t\tVoter_out Constructor");
this.voter = voter;
System.out.println("\t\tThread name : " + Thread.currentThread().getName());
}
public void run()
{
int timer = (int)(Math.random()* 100);
System.out.println("\t\tThread name void run : " +
Thread.currentThread().getName());
for(int i = 1; i < 5; i++)
{
try {
//voter.voterout(i);
System.out.println("\t\tVoter Voted " + voter.voterout(i));
System.out.println("\t\t** name : " + Thread.currentThread().getName());
Thread.sleep(timer);
}
catch(InterruptedException e)
{
System.out.println("\t\tlost track of voter" + e);
}
}
}
}
|
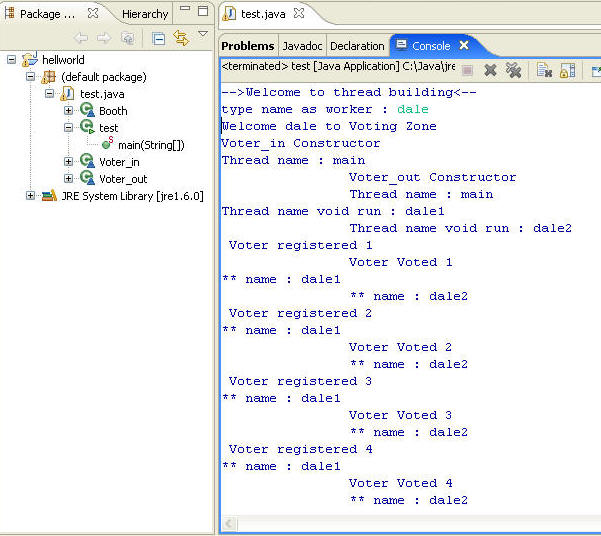 |
|