Step: Brief discussion:
Prototype: void cin.getline(char *ch1, const int size);
In the previous examples, namely
cin_stream1.htm and
gets_unsafe1.htm,
we understood the error handling schemes of iostream libraries of
C++, especially handling stack-buffer overflow.
Some functions are globally persistent, and others are volatile,
meaning that these functions work as tributaries to the persisted
functions.
Here in this example
we took a closer look at ""cin.fail()" function.
Create another source file, as shown below. The first illustration
shows the code snippet in main function, and second illustrations
shows the code snippet of "cinchcks" function.
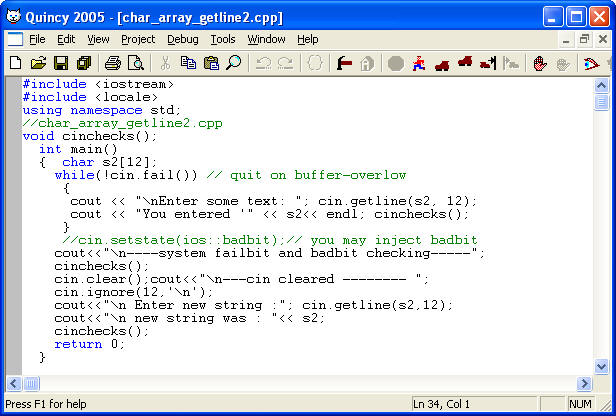
The codes in cinchecks function.
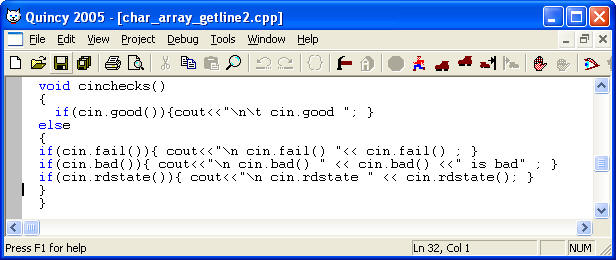
Run this application and type a short string to avoid
buffer overflow. Then at the next prompt enter an oversized string,
as indicated below.
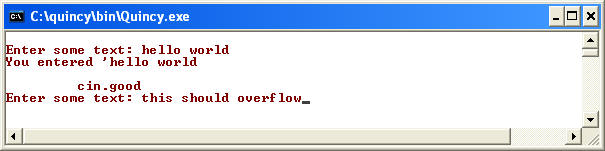
The oversized data raised a fail flag, but the data was not bad.
The error could be cleared
out with "cin.clear" function. The function "cin.ignore",
used in the above example, would ignore the stream (emptying the buffer)
and prompt
another chance to the user to enter another string.
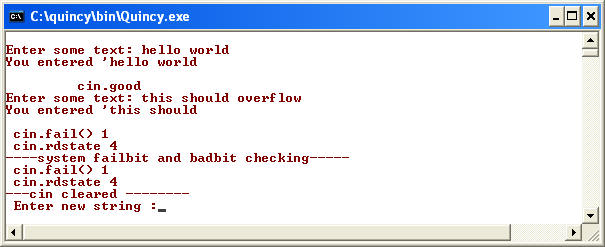
As long as the user, enters correct size of data, there won't be any
error .
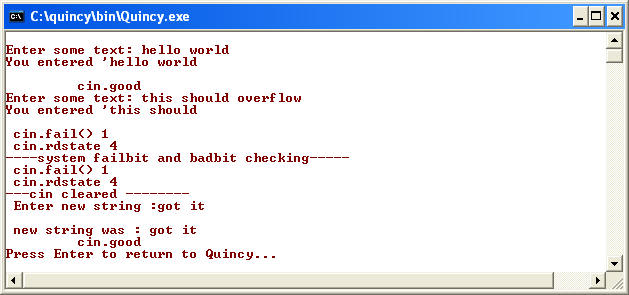
The function, cin.getline(cs1, 12), extracts characters from the input sequence and stores
them as a c-style-string into the array and terminated by a null
character. If the user enters more than size-1 characters, the
function raises flag, "failbit" and terminate the operation. This
will prevent the system from holding up or crash.
It appears that cin.fail() is a whistle blower of iostream, and
raises the flag once with a cin.failbit, and cin.badbit. The flag,
failbit, is raised from an internal error, can be cleared up for
succeeding operations. But the flag, "badbit" is only raised due to
bad-stream or corrupted stream, and suspends other
operations. Please visit (ios_failbit1.htm
)