Step: Brief Discussion:
This example shows the risks involved in using "gets(parameter)"
function, and these risks can be eliminated with dynamic streaming
with iostream. The iostream schemes are empowered with cin and cout
objects, and an efficient error handling routines. The cin object
suspends input-streaming in case of any error, thereby preventing an
application from crashing (Please refer
cin_stream1.htm).
As C++ introduced streaming in real sense and terms (
iostream_cin_cout1.htm ), the function "gets(string)", is a step
back towards the C-string concepts. Please review this example,
where I used a string object to get user's input from key board, and
then initialized a character array.
char_new_delete1.htm.
Unsafe gets(array): The
function, get() of , is not safe and can misbehave.
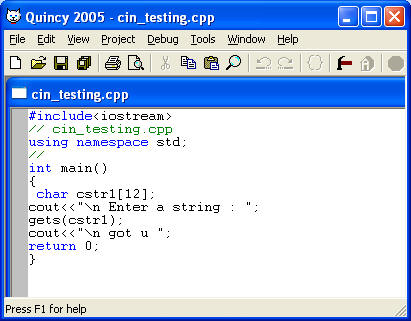
An application may crash with gets(array) function: Since
gets function does not define size limit and does not have error
handling supports; the users can enter a very long string and that
may crash the application, as shown in the illustration below.
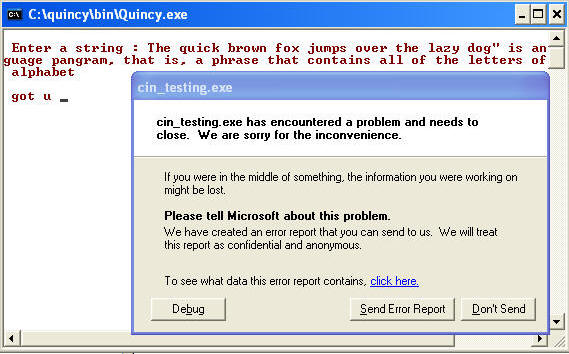
gets(...) function can misbehave: In some IDE, like Eclipse
CDT, gets()
function did not blend along with objects from iostream. In
the following example, compiler did not process it's (gets()
function) instructions. ( You need to tune up your Eclipse software
to support C99 I found a nice hands on article
http://bitvector.net/?p=75)
. I tested in windows 32 and 64 bit OS, most of the old C codes
worked, but strolled with gets(..) function).
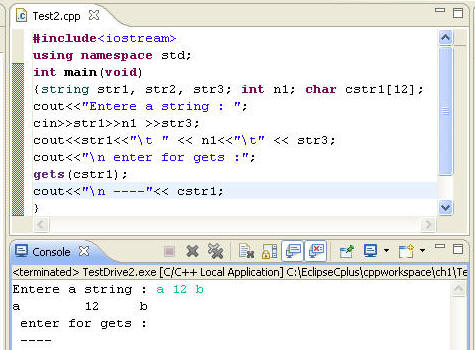
cin object and buffer-overflow :
In this example, the function
"cin.getline(cstr1, 12)", contains an array with predefined storage
size. An array stores homogenous data, and the size of the array
must be declared before using. The reference of fixed size arrays
are stored in an area known as "stack".
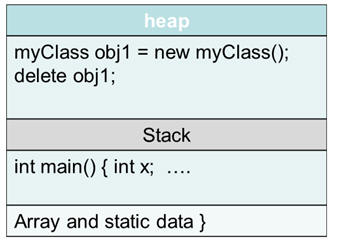
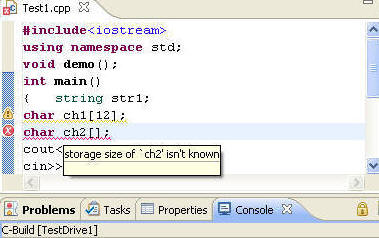
The stack is a storage area, and is used to
store data declared implicitly (pre-defined). The data is
stored in stack using the Last In First Out (LIFO) method. The array elements are stored
in the stack and the compiler will verify the size allocated for
this array. When you feed a string which
exceeds the allocated size, compiler will fail to process the
oversized-string, and cause buffer-overflow.
A buffer overflow, as we see in one of the
illustrations, is an anomaly where the input streams overruns the
allocated space. This may cause many unexpected results, erratic
application behavior, unexpected output, violate security rules or a
crash. The error handling schemes of isostream, recognizes
such errors, enters the a fail-state, and ignores all references to
input-stream operations. C language does not provide such
operations.