The following example shows how to Overloading Methods in a class than
Overriding Methods using many extended classes. |
|
see also
Link |
Overloading methods. |
Overriding method. |
|
- An overriding method replaces the method
contents keeping the format as is declared in an abstract class and
then overrides the contents.
- The rules in the first generation is strict, however
loose in the derived classes.
- An overriding method in the first generation must have argument lists of identical
type and order.
- The return type must be same as in the first generation that of overridden method.
- Example
class A extends An_abstractclass{
public String method_1(String str)
{--do something--- }
class B extends An_abstractclass{
public String method_1(String str)
{--do something else--- }
abstract An_abstractclass
{
public String method_1(String str);
}
|
|
Overriding (re Write as it is from on line )
- When a class defines a method with same method name, argument types,
argument order and return type as a method in its super class, its called
method overriding.
- The method in the super class is said to be overridden by the method
in the subclass.
- Overriding method actually replaces the behavior in super class for a
subclass.
- Rules for overriding
- Methods overriding cannot be declared more private than the super
class method.
- Any exceptions declared in overriding method must be of the same
type as those thrown by the super class, or a subclass of that type.
- Methods declared as final cannot
be overridden.
- An overriding method can be declared as
final as the keyword final only suggests that this method cannot
be further overridden.
- Methods declared as private cannot
be overridden as they are not visible outside the class.
- Overriding method can call the overridden method (just like any other
method) in its super class with keyword super.
The call super.method() will invoke the
method of immediate super class.
- Though keyword super is used to
refer super class, method call super.super.method() is invalid.
|
Overloading (re Write as it is from on
line )
- If two (or more) methods of a class (whether both declared in the same
class, or both inherited by a class, or one declared and one inherited)
have the same name but different signatures, then the method name is said
to be overloaded.
- The signature of a method consists of the name of the method and the
number and types of formal parameters in particular order. A class must
not declare two methods with the same signature, or a compile-time error
occurs.
- Only the method name is reused in overloading, so method overloading
is actually method name overloading.
- Overloaded methods may have arguments with different types and order
of the arguments may be different.
- Overloaded methods are not required to have the same return type or
the list of thrown exceptions.
- Overloading is particularly used while implementing several methods
that implement similar behavior but for different data types.
- Overloaded methods are independent methods of a class and can call
each other just like any other method.
- Constructor Overloading
- Constructors can also be overloaded in similar fashion, as they are
also methods.
- Java has some classes with overloaded constructors. For example,
wrapper class for int has two
overloaded constructors. One constructor takes
int as argument and another takes
string argument.
Integer aNumber = new Integer(2);
Integer anotherNumber = new Integer("2005");
- One constructor can call another overloaded constructor of the same
class by using this keyword.
- One constructor can call constructor of its super class by using the
super keyword.
|
import java.io.*;
import java.lang.reflect.*;
public class Test_This {
public static void main(String[] args) throws IOException{
// TODO Auto-generated method stub
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
String str ="", str1="";int n1=0, n2 = 1;
Process pp = new Process();//object is created
Class gt = pp.getClass();
Method[] mt = gt.getMethods();
try{
System.out.print("Please write your name: ");
str = br.readLine();
if(pp.getClass()== Process.class){
System.out.println("Below cames from class : " + pp.getClass().getName());
System.out.println(pp.method_function(str)); //calling this method using
object pp
System.out.println("\tmethod type : " + mt[1].getReturnType().getName());
System.out.print("Please write your age: ");
str = br.readLine();
n1 = Integer.parseInt(str);
System.out.println("\tmethod type : " + mt[2].getReturnType().getName());
System.out.println(pp.method_function(n1)); //calling this method using
object pp
}
}//try
catch(NumberFormatException e)
{ System.out.println("data was blank");}
}
}
class Process
{
//class body
public int n1 =12; String name = "";//defined and instantiated a filed
public String method_function(String str)
{
//function body
name += str.toUpperCase();
System.out.print("Welcome : " );
return name;
}
public String method_function(int str)
{
//function body
if (str<=20)
{
name += " you look like 16 than " + str;
}
else
{
name += "you are under age :";
}
System.out.println("you entered : " + str);
return name;
}
}
|
Overloading Methods, meaning same name signature with different
parameter types or return type. |
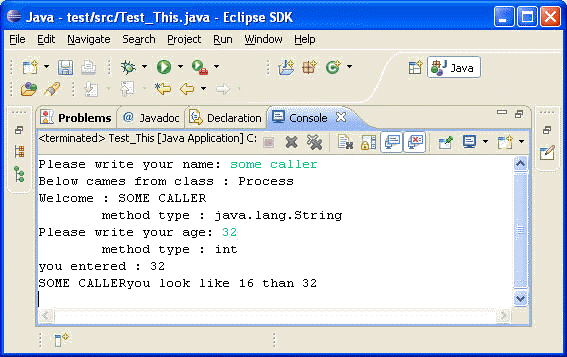 |
I believe the above example has a twitch, method parameter also depends
on the user's argument, if you have functions overloaded, the parameter type
should differ, typing identical parameter will conflict with each other, the
sender will not know which of the twin to get this data. |
|
Similar string parameter, with different method types, like String an
int method types respectively.
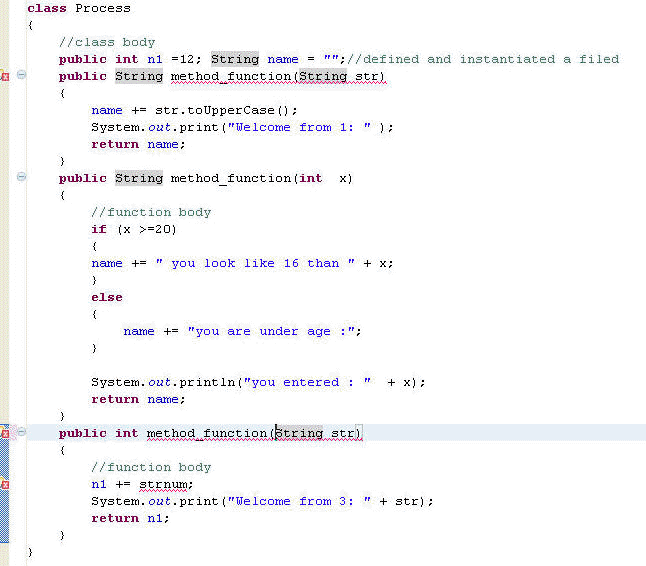 |
OR |
|
Here Integer parameter type conflicted with each other.
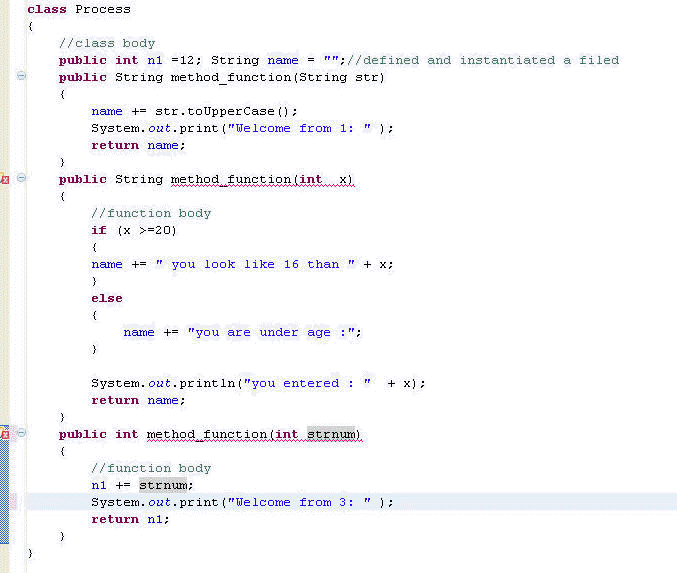 |
This is OK, when you different parameter types, now Java will know where
to send |
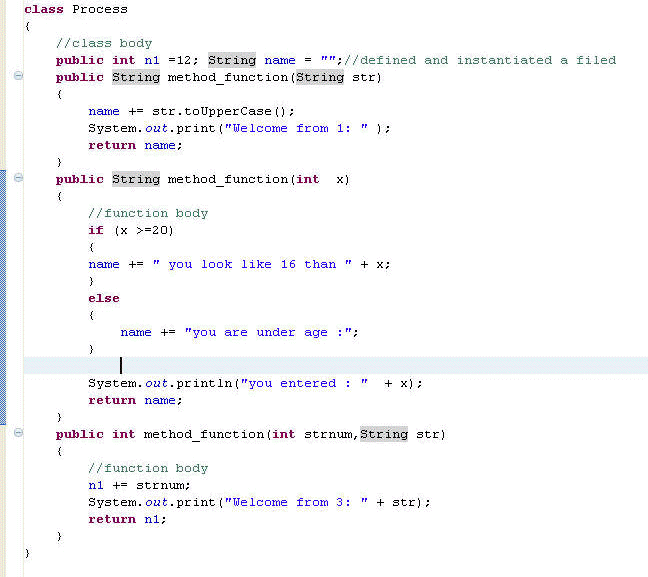 |
|
Example 2: |
import java.io.*;
import java.lang.reflect.*;
public class Test_This {
public static void main(String[] args) throws IOException{
// TODO Auto-generated method stub
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
String str ="", str1="";int n1=0, n2 = 1;
Process pp = new Process();//object is created
Class gt = pp.getClass();
Method[] mt = gt.getMethods();
try{
System.out.print("Please write your name: ");
str = br.readLine();
if(pp.getClass()== Process.class){
System.out.println("Below cames from class : " + pp.getClass().getName());
System.out.println(pp.method_function(str)); //calling this method using
object pp
System.out.println("\tmethod type : " + mt[1].getReturnType().getName());
System.out.print("Please write your age: ");
str1 = br.readLine();
n1 = Integer.parseInt(str1);
System.out.println("\tmethod type : " + mt[2].getReturnType().getName());
System.out.println(pp.method_function(n1)); //calling this method using
object pp
//
System.out.println("\tmethod type : " + mt[3].getReturnType().getName());
System.out.println(pp.method_function(n1, str)); //calling this method
using object pp
}
}//try
catch(NumberFormatException e)
{ System.out.println("data was blank");}
}
}
class Process
{
//class body
public int n1 =12; String name = "";//defined and instantiated a filed
public String method_function(String str)
{
name += str.toUpperCase();
System.out.print("Welcome from 1: " );
return name;
}
public String method_function(int x)
{
//function body
if (x >=20)
{
name += " you look like 16 than " + x;
}
else
{
name += "you are under age :";
}
System.out.println("you entered : " + x);
return name;
}
public int method_function(int strnum,String str)
{
//function body
n1 += strnum;
System.out.print("Welcome from 3: " + str);
return n1;
}
}
|
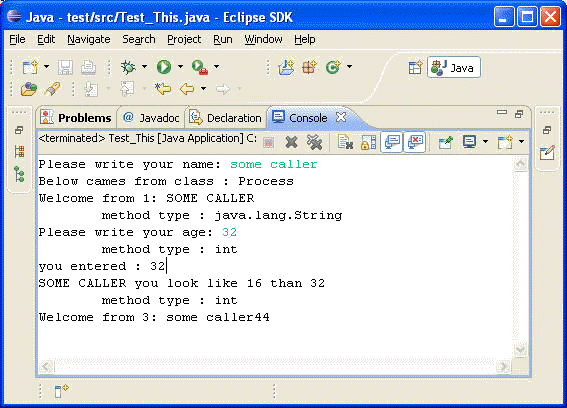 |
|