Class Basics: |
Also view this
page. |
Below is the bare minimum requirement to create a
class and object |
Defining a class
(default private) class Class_name //declaring class name {
//class body starts within these two braces
//constants, method, fields,
}
public (accessor) class Entry_point //this is required {
Class_name cn = new Class_name(); // instance of a class and object created with new keyword
}
|
|
Inheritance:
(public or private) class derived_class extends super_class implements
custome_interface
{
//field, constructor, and method declarations
}
|
|
derived class is like a
child class, can inherit from only one caring parent class
(public or private) class derived_class extends super_class implements
custom_interface
{
//field, constructor, and method declarations private class_nested
{
my_parent.my_self my_object = new my_parent.my_self my();
}
}
|
|
Inner class:
There are two additional types of inner classes.
Local and Anonymous Inner Classes
You can declare an inner class within the body of a method.
public class class_name
{ public void a_method()
{
class A_local { //code implementation
}
}
}
Such a class is known as a local inner class.
You can also declare an inner class within the body of a method without
naming it.
These classes are known as anonymous inner classes.
Anonymous inner clases
class Process {
public Process()
{
System.out.println("Process outer constructor");
}
public int n2; String name="";
public void a_method(String str_method)
{
//contents of anonymous
{
class name = this.getClass().getName();
System.out.println("Received at anonymous inner class" + str_method);
}
//end of anonymous class
System.out.println("Retreive class name at inner class: " + name);
} //end method_1
}//end of class process
|
|
Class accessor Modifiers, Fields/Variable, Method:private, public and abstract, if a class is not
specified, default is private.
- fields : that indicates the type of data is being hold by an
object. Also know as instance variable
- constructor : keeps track of the object and evoked when an
object (new instances of class using new key word) is instantiated. The
constructors are created just like other method, but it is named after
the class.
- class-name Do_something(); // declaration
- public Do_something() { // no parameter
}
- public Do_something(String str, int n1) { // with two parameter to
handle two arguments from a caller function }
}
- method : process client's request. One method can call
another with some rules and whether these method would be accessible out
side of the class or not, is determined by the accessor keywords
(access modifiers) like public, protected, private, final or
abstract.
- public methods are accessible with object created in the
client's routine, then makes a request using appropriate syntaxes.
- private: If a class is not
|
creating an object
- One Line with no argument/parameter pair.
- Class-Name a-variable = new Class-Name();
- One Line with one argument/parameter pair.
- Class-Name a-variable = new Class-Name(feed-argumet-to-a-parameter);
|
import java.io.*;
// javac simple_client_1.java
public class simple_client_1
{
public static void main(String args[])throws IOException
{
System.out.println("Client Starts");
//creating object where ss is reference to the class simple server_1
simple_server_1 ss = new simple_server_1();
ss.process();
System.out.println("Client ends");
}//end of main
}//end of class
class simple_server_1
{
//constructor
public simple_server_1(){ System.out.println("Server Constructor Starts as
an object is created");}
//these are the fileds
private int n1 = 0;
private int n2 = 0;
public String str2="Call Me if you need";
//method starts
//accesor type public
//return type void
//() place holder for parameters
public void process() throws IOException
{
//implementation with code satrs
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
////////////////
String str= "";
try {
System.out.println("---While Loop---");
while(( n1 !=9)&&(n2 !=11) )
{
System.out.print("Please enter number: ");
str = br.readLine();
int n3 = Integer.parseInt(str);
n1 = n3;
System.out.print("Please enter pin: ");
str= br.readLine();
int n4=Integer.parseInt(str);
n2= n4;
System.out.println("You Entered : " + n1 + n2);
}
}//try
catch(NumberFormatException e) { System.out.println("data was blank");}
///
System.out.println();
}//end of main
}//end of class |
When you compile, the code creates two classes that java virtual machine
will look during the run time. |
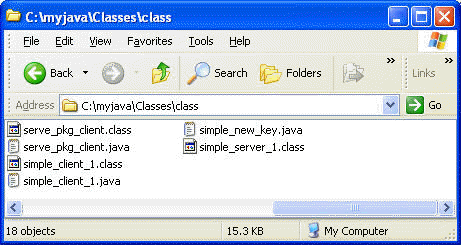 |
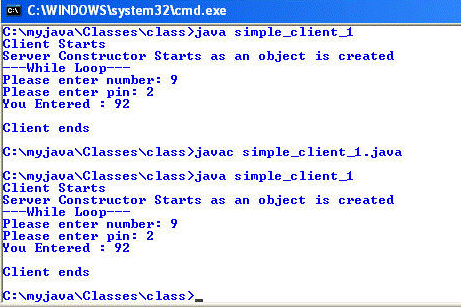 |
|
|
|
|
|