|
Inheritance: Inheriting parent's properties, all tags and attributes are
packaged for your use.
- privileges are regulated by the parent or base or super class,
these names are synonymous.
|
Step :1 The code below shows how to create super class, and
the scope of the members. |
class A
{
public int share_all = 12;
private int share_within = 24;
protected int share_protedted = 48;
public A()
{
System.out.println("Base/Super class A ");
}
public void method_share()
{
}
protected void method_protected()
{
}
private void method_private()
{
int n1 = 20;
}
}
|
Step 1-Images This symbols are used in Eclipse GUI to
compile java codes.
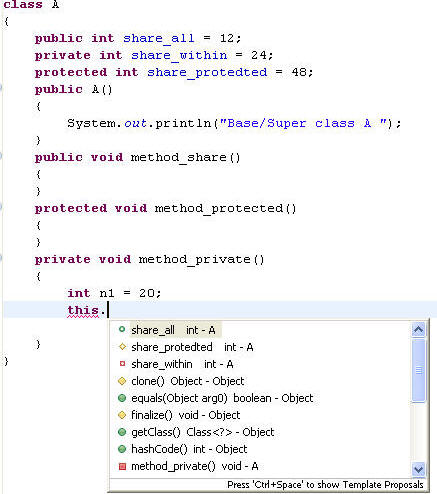 |
Step 2- Images : Edit the existing code: Note the scope of
private field member
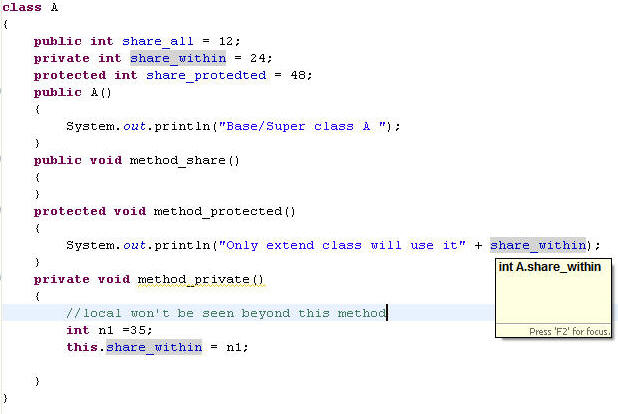 |
Step 3: Create class B, and add the following codes |
class B extends A
{
public B()
{
System.out.println("Extended class B Constructor ");
}
}
|
The complete code of super class A and extended class B. |
class A
{
public int share_all = 12;
private int share_within = 24;
protected int share_protedted = 48;
public A()
{
System.out.println("Base/Super class A ");
}
public void method_share()
{
}
protected void method_protected()
{
System.out.println("Only extend class will use it" + share_within);
}
private void method_private()
{
//local won't be seen beyond this method
int n1 =35;
this.share_within = n1;
}
}
class B extends A
{
public B()
{
System.out.println("Extended class B Constructor ");
}
}
|
Step 4 : calling the classes. the green letters shows how to
create an instance of one class in another class with new Key word. But
object is not formed. If you run this code in Eclipse |
public class test
{
String str = "Helloworld";
public static void main(String[] args) throws IOException
{
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
// needs throws IOException
System.out.print("Type some thing : ");
String str =br.readLine();
B b ;
}
}
|
Now, if you call this instance of B class, you would notice
that none of the extern classes (A or B), were engaged.
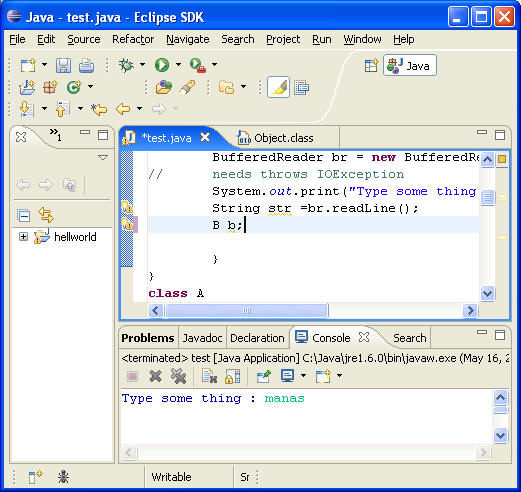 |
Now watch the code, I created an object using new Keyword.
Instance of class and object of a class can be created in one line, as shown
below.
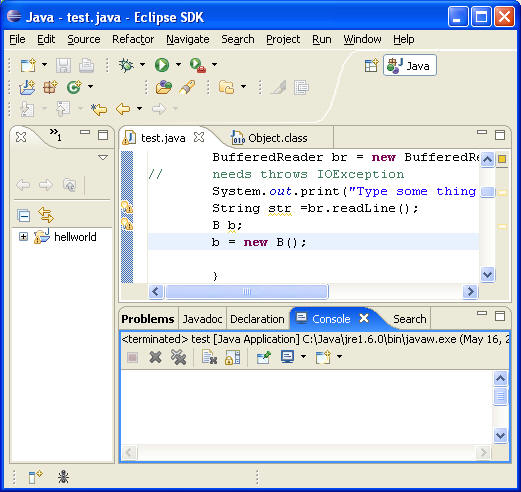
|
Notice the difference in the out put generated when we ran
this application. |
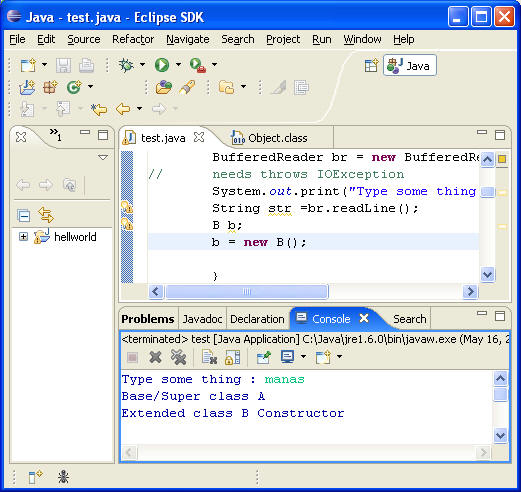 |
Once the the object from a class created, you are allowed to
use the inherited fields and methods members, those are declared as either
protected or public. The private members in the base class can't be accessed
from the derived class. |
|
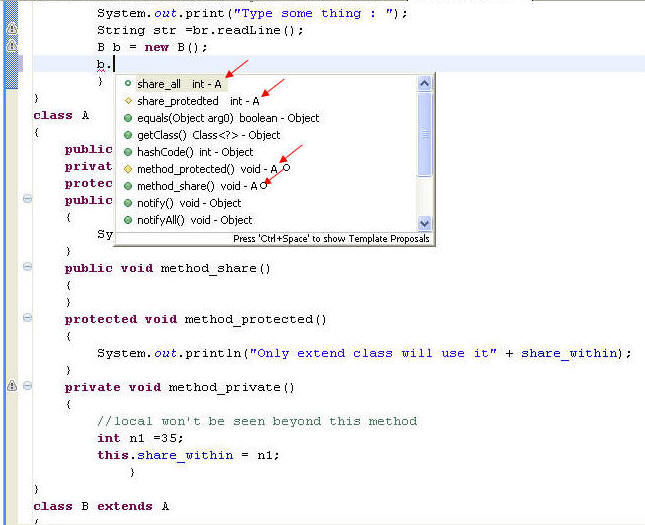 |
The completed routine
import java.io.*;
import java.io.IOException;
//javac clas_name.java
public class test
{
String str = "Helloworld";
public static void main(String[] args) throws IOException
{
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
// needs throws IOException
System.out.print("Type some thing : ");
String str =br.readLine();
B b = new B();
System.out.println("The value from Base via derived class :"+ b.share_all);
System.out.println("The value from Base via derived class :"+
b.share_protected);
b.method_protected();
b.method_share();
}
}
class A
{
public int share_all = 12 ;
private int share_within = 24;
protected int share_protected = 48;
public A()
{
System.out.println("Base/Super class A Constructor");
}
public void method_share()
{
}
protected void method_protected()
{
System.out.println("Only extend class will use it" + share_within);
}
private void method_private()
{
//local won't be seen beyond this method
int n1 =35;
this.share_within = n1;
}
}
class B extends A
{
public B()
{
System.out.println("Extended class B Constructor ");
}
}
|
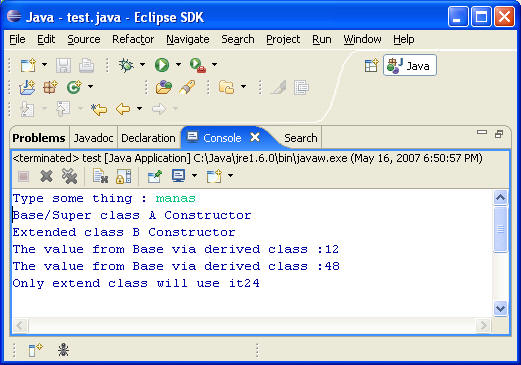 |
In the above example Class B inherited all the methods, but
never used those methods. To force a method or few methods in the derived
class, you can make an abstract base class . The use of abstract class is
explained in another example.
Link |