- Objects :
Connection c = null; Statement st = null;
Properties param = new Properties();
- Connect DB : One string connects db with users name and pwd
c = DriverManager
.getConnection("jdbc:postgresql://localhost:5432/postgres",
"postgres", "postgre_manas9");
OR
c = DriverManager.getConnection("jdbc:mysql://localhost:5432/test?","Manas9",
"Manas9237");
- Properties: user and pwd are tendered by
variables/literals
param.put("user",user); param.put("password",pwd);
c = DriverManager.getConnection(dbURL,param);
To avoid hard coding all the database parameters in the code, you
can use a Java properties file to store them. In case of changes,
you just need to change them in the properties file and you don’t
have to recompile the code.
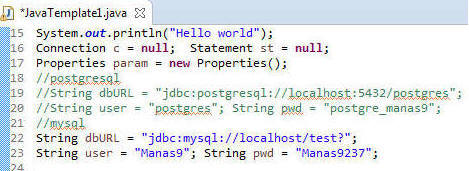
|