TreeSet1.htm |
- Collection :: represents a group of ELEMENTS, and is the root of
collection hierarchy
- Set :: can't have duplicate element
- List:: ordered collection, can have duplicate elements
- Queue: holds multiple elements, provides additional insertion,
extraction and inspection, it support FIFO
- Deque:: support FIFO and LIFO, elements can be
added/removed at the both end
|
- List, Stack, Queues are considered to be linear data structure.
- A TreeSet is a non-linear two dimensional data structures
|
Interfaces |
Implementations |
|
Hash table |
Resizable array |
Tree |
Linked list |
Hash table + Linked list |
Set |
HashSet |
|
TreeSet |
|
LinkedHashSet |
List |
|
ArrayList |
|
LinkedList |
|
Queue |
|
|
|
|
|
Map |
HashMap |
|
TreeMap |
|
LinkedHashMap |
|
- TreeSet tender an implementation of Set interface , the object
are stored in ascending order
- Constructors:
- TreeSet( )
- TreeSet(Collection C )
- TreeSet( Comparator comp)
- TreeSet( SortedSet s)
|
Example of tree 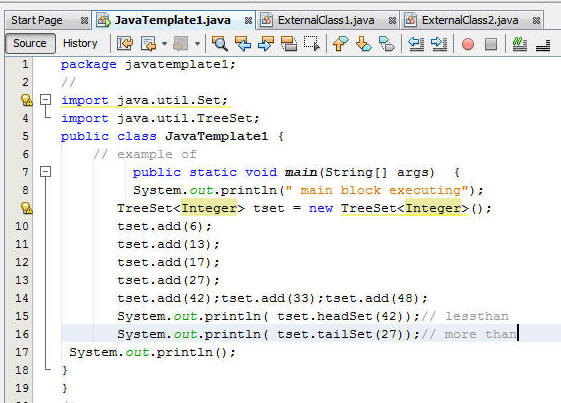
Run Time View
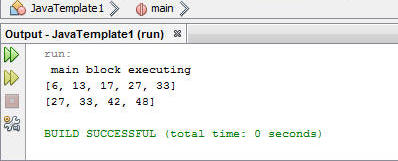
|
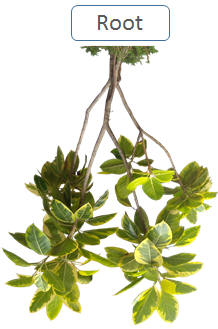 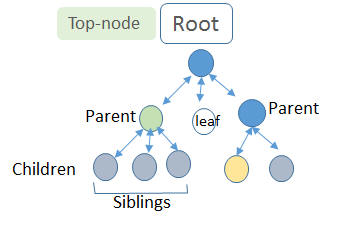 |
- Just like Linked Lists, Trees are collections of nodes
- Orientation of a Tree is upside down (like family trees)
- The top node is the root
- nodes are connected by edges
- edges (arrows) define parent and child nodes
relationships
- nodes with no children are called leaves
|
- binary tree:
A tree in which each node refers to 0, 1, or 2 dependent nodes.
- root
The top-most node in a tree, to which no other nodes refer.
- leaf
A bottom-most node in a tree, which refers to no other nodes.
- parent
The preceding node that refers to a given succeeding node.
- child
One of the derived /succeeding nodes referred to by a node.
- level
The set of nodes equidistant from the root.
- prefix notation
A way of writing a mathematical expression with each operator
appearing before its operands.
- preorder
A way to traverse a tree, visiting each node before its children.
- postorder
A way to traverse a tree, visiting the children of each node before
the node itself.
- inorder :A way to traverse a tree, visiting the left subtree, then the root,
then the right subtree.
- class variable
A static variable declared outside of any method. It is accessible
from any method.
- binary operator
An operator that takes two operands.
- object encapsulation
The design goal of keeping the implementations of two objects as
separate as possible. Neither class should have to know the details
of the implementation of the other.
- method encapsulation
The design goal of keeping the interface of a method separate from
the details of its implementation.
|
Path and siblings: A Path or edge is a sequence (hierarchies) of nodes, a
parent node tender child nodes, and nodes under a parent-node are
siblings,
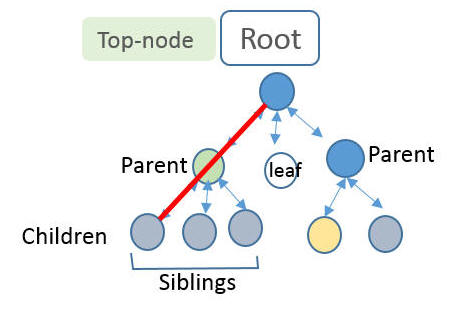
|
The depth of a node is the length of the path from the root, and
height of a tree is the maximum depth, meaning the length of the path
from the root to the last node.
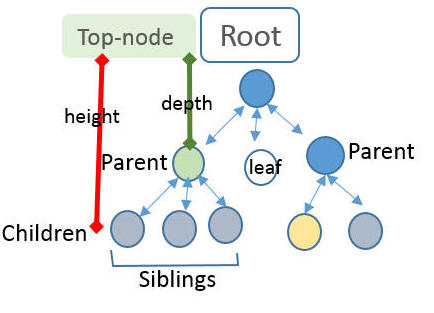
|
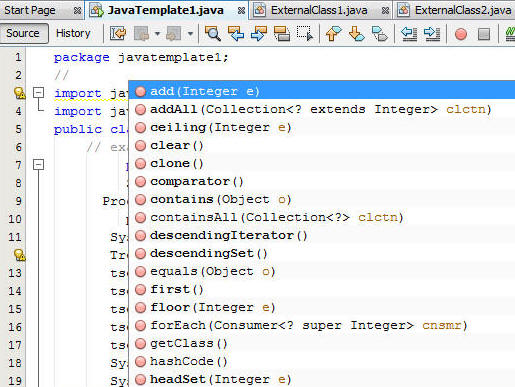
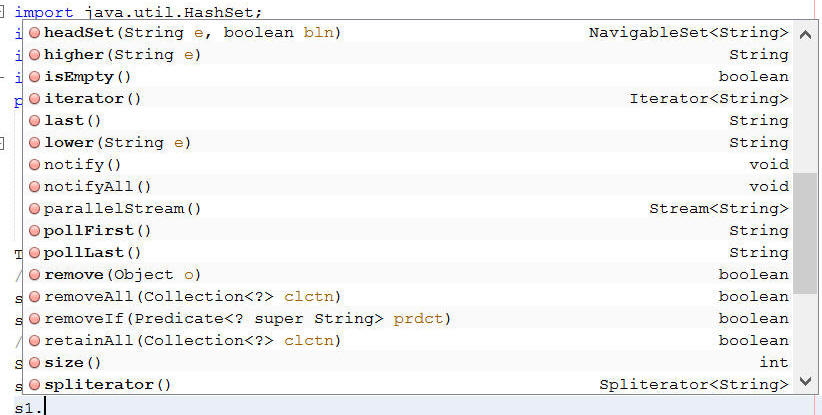
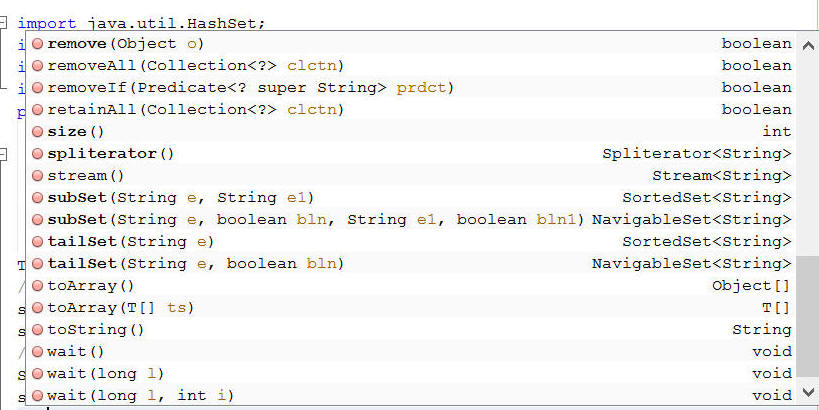
|
Code Used :
package javatemplate1;
import java.util.Set;
import java.util.TreeSet;
public class JavaTemplate1 {
// example of
public static void main(String[] args) {
System.out.println(" main block executing");
TreeSet<Integer> tset = new TreeSet<Integer>();
tset.add(6);
tset.add(13);
tset.add(17);
tset.add(27);
tset.add(42);tset.add(33);tset.add(48);
System.out.println( tset.headSet(42));// lessthan
System.out.println( tset.tailSet(27));// more than
System.out.println();
}
}
|
|
|