Creating Objects: (continued) |
- new operator is commonly used to
create an object
- Sting does not create an object,
- Code
String title = " enclosed in a double quotes" ;
|
Now this "title", as it represents String object, it will inherit all
sate and behaviors (method and properties).
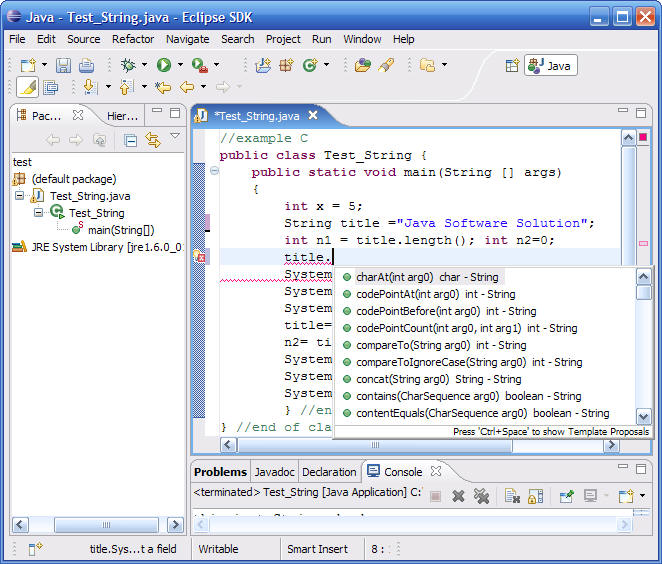
|
Read more about methods and constructor: (Link)
- Once a String object created, it's value and length can't be changed
- String class is immutable
- The contents of string literals are indexed starting from 0. In a
string "Hello" , "o" is indexed at 4.
|
|
String is Indexed.
|
|
|
String methods are useful, especially to a return a number or a string
and display as an out put. as show below ( look advance concept right now,
trust me it is not).
|
//javac test_get_set.java my code
// This is your Base class
public class test_get_set
{
//declaring variable
private int player_no;
public test_get_set (){ System.out.println("This is a constructor with no
param");}
public static void main(String[] args) throws IOException
{
// creating instance and object "t" of the test class
test_get_set t = new test_get_set();
//send an argument to a method
t.set_no(12);
// ca
System.out.println("Player no : " +t.get_no());
}
// This is a private method to this method returns an integer when
you call
private int get_no()
{
System.out.println("This method returns value to the calling function : "
);
//intializing variable playr_no
player_no = 12;
return player_no;
}
//This method will consume the input but won't return any value
//as return is blocked
private void set_no(int n1)
{
System.out.println("This method setting a value of a variable : " );
//intializing variable playr_no
player_no = n1;
//return player_no;
}
}//end of the class
|
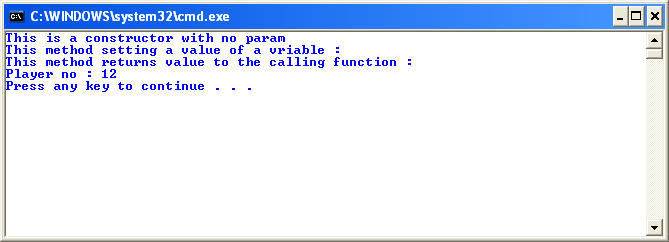
|
Strings are mutable or immutable ? Looks like mutable, but actually
it is not mutable. It would have been easy to demonstrate to prove
immutability, if java had addressOf operation or pointer in java.
public class Test_String {
public static void main(String [] args)
{
int x = 5;
char data[] = {'a', 'b', 'c'};
String str = new String(data);
//java claims that Strings are not mutable, but it is
// note str object can change itself by calling itself with a new string
String title = str + str.replace(str, "cba");
int n1 = title.length(); int n2=0;
System.out.println("this is toString:"+ title.toString());
System.out.println("title.length();" + n1);
System.out.println("title.charAt(0);" + title.charAt(0));
title= "New value";
n2= title.length();
System.out.println("this is toString:"+ title.toString());
System.out.println("title.length();" + n2);
System.out.println("title.charAt(0);" + title.charAt(0));
} //end of the main
} //end of class
|
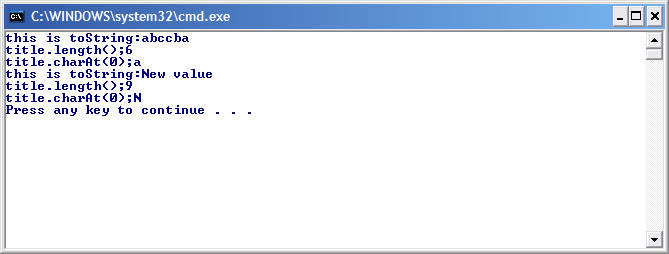
|
//csc StringsRImmutable.java
public class StringsRImmutable
{
public static void main(String[] args)
{
String one = "Lake Park HighSchool";
String two = new String("Lake Park HighSchool");
System.out.println("String Immutable) ?--");
System.out.println(one.equals(two));
System.out.println(one == two);
two = one;
System.out.println(one == two);
}
}
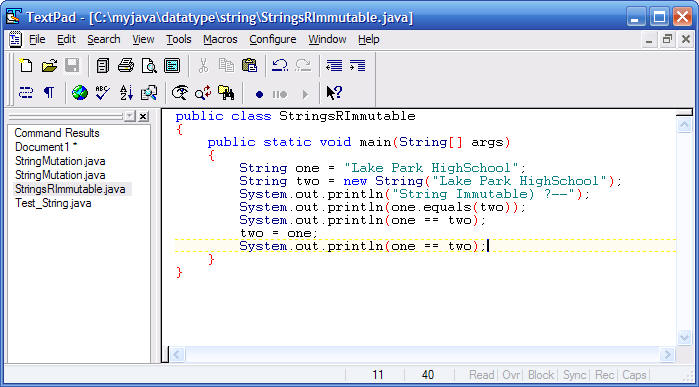
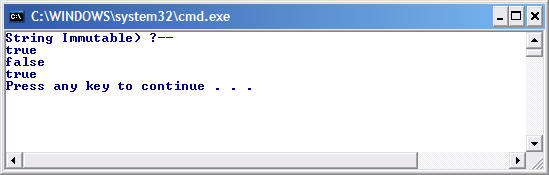
|
|
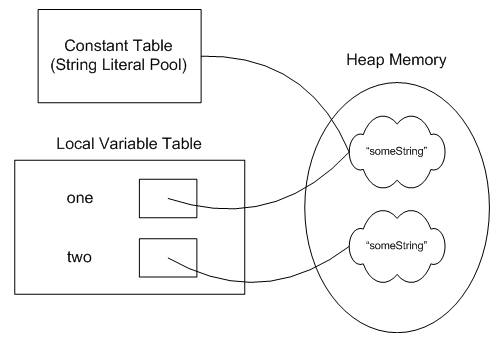
Can I prove it in Java? Not yet, Java does not have pointer, like C++
or VB.Net , or C#'s delegates
|
very close to prove it
//csc StringsRImmutablehasCode.java
public class StringsRImmutablehasCode {
public static void main(String[] args)
{
int x = 5;
String title ="Java Software Solution";
int n1 = title.length(); int n2=0;
System.out.println("this is toString:"+ title.toString());
System.out.println("title.length();" + n1);
System.out.println("title.charAt(0);" + title.charAt(0));
System.out.println("this is hashCode():"+ title.hashCode());
title= "New value";
n2= title.length();
System.out.println("this is toString:"+ title.toString());
System.out.println("title.length();" + n2);
System.out.println("title.charAt(0);" + title.charAt(0));
System.out.println("this is hashCode():"+ title.hashCode());
}
} //end of class
|
Although we were thinking we were mutating, but did not
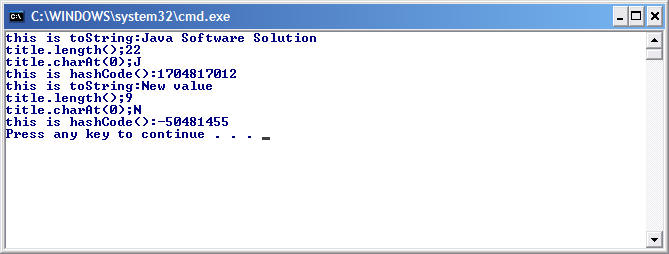
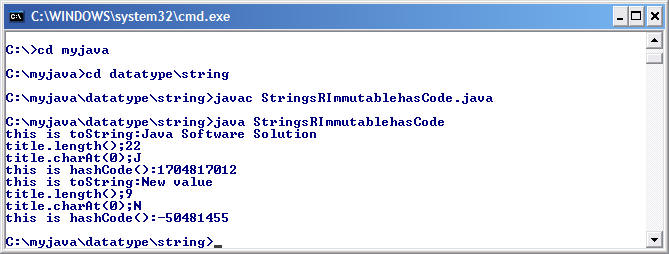
|
|
|