This an example of using nested loop depending on the feed from parent
loop.
- Nested loop will complete first before sending the handle to parent
loop;
- unless, nested loop has an check back or exit condition that checks
some parameter in the parent loop. Set -1 and set -2 are an example of
that condition.
- However, the above condition was created with either
<= or < operator. With <= operator you let the nested loop run when
value of a local variable is less than or equal to the value of
a variable in parent.
- In set three, run condition in the nested loop is independent of
parent loop( z = 4); therefore the loop with run four times multiplying
(i * z ) for time times, the print out will depend on the value of
i in the parent loop
|
import java.io.*;
// javac test_for_b.java
public class test_for_b
{
public static void main(String args[])throws IOException
{
//////////////////////////////////////
System.out.println("--set 1--");
for(int i = 1; i < 5 ; i++)
{
System.out.println("\t round start --: " + i);
for(int z = 0; z <= i; z++)
{
System.out.print(i * z + " : ");
}
System.out.println();
System.out.println("\t round end --: " + i);
}
/////////////////////////////////////
System.out.println("--set 2--");
for(int i = 1; i < 5 ; i++)
{
System.out.println("\t round --: " + i);
for(int z = 0; z < i; z++)
{
System.out.print(i * z + " : ");
}
System.out.println();
System.out.println("\t round end --: " + i);
}
////////////////////////////////////
System.out.println();
System.out.println("--set 3--");
for(int i = 1; i < 5; i++)
{
System.out.println("\t round --: " + i);
for(int z = 0; z <= 4; z++)
{
System.out.print(i * z + " : ");
}
System.out.println();
System.out.println("\t round end --: " + i);
}
///////////////////////////////////
}
}
|
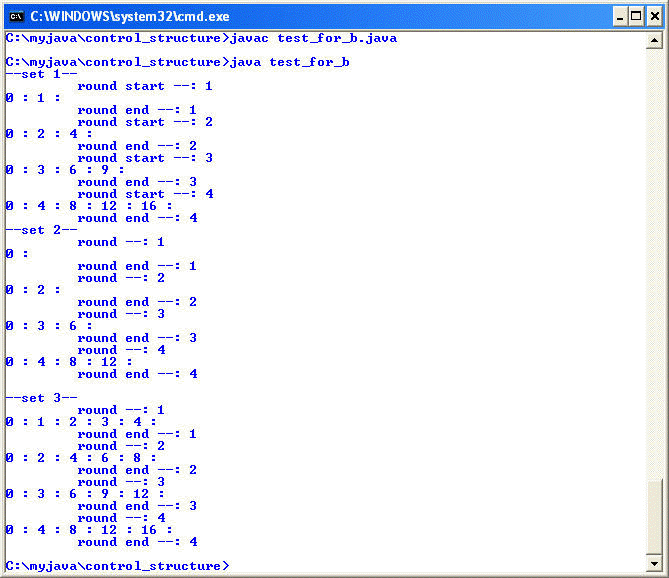 |
Using another compiler C# I want to show the flow of this routine.
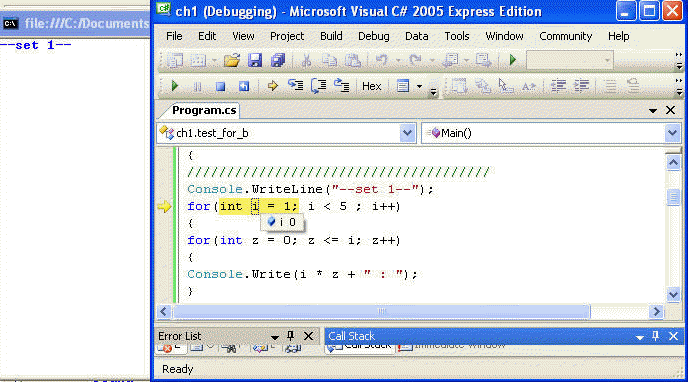 |
next step compiler verifies the limit of the routine,
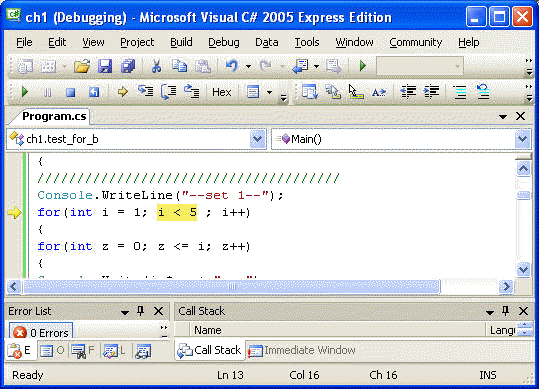
|
Step 2: debugging, at this point compiler enters the inner for loop,
here condition is <= , meaning, if a local variable z is less or equal
(z <= i;) to a public variable i
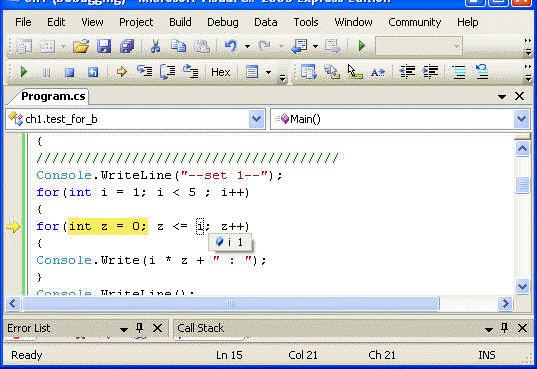
The illustration shows a condition where value of z = 0, when i= 1;
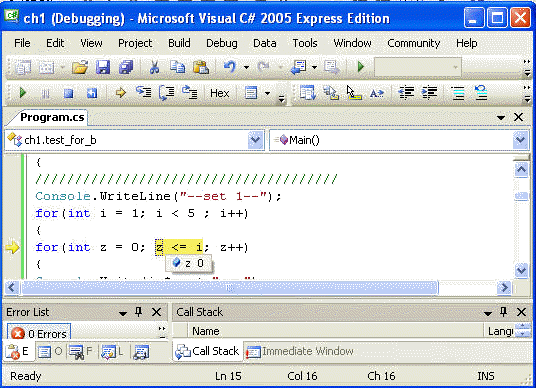
|
Step 3: Calculates ( i * z ) = (1 * 0 = 0);
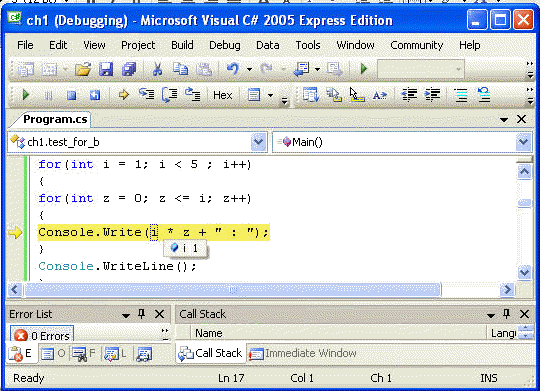
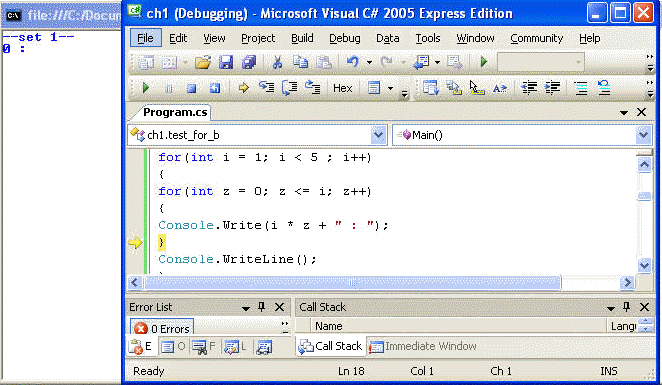 |
Step :4 going back to inner loop
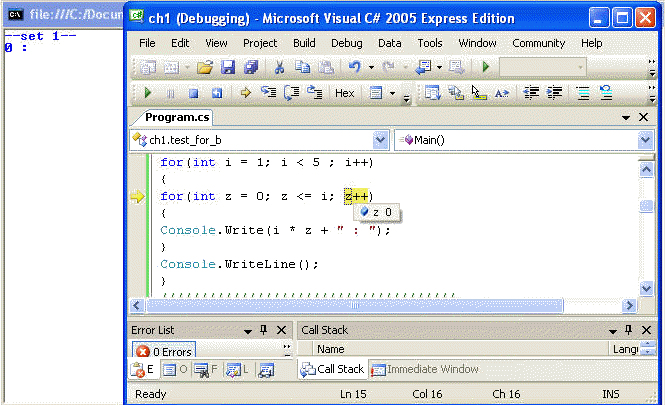
And finds the value of z is 1 and that is still equal to the current value
of i ( i= 1);
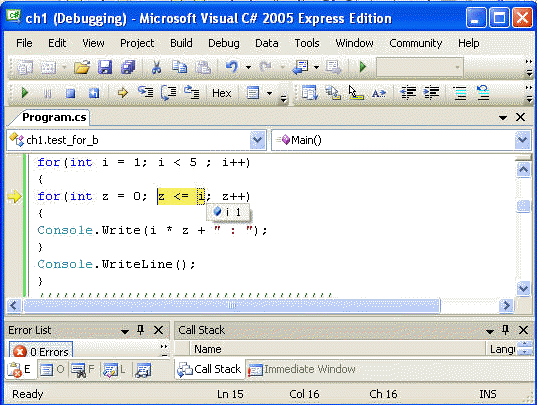
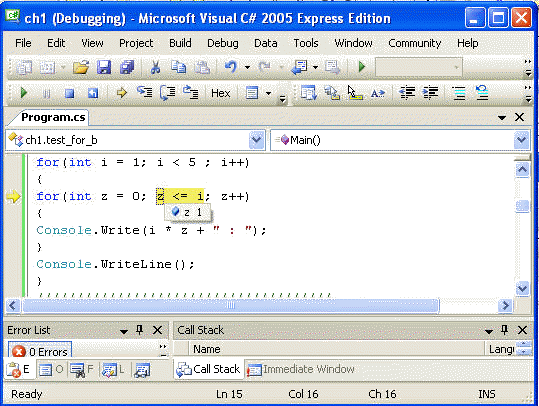
|
Step 5: At this step, in the second round in the inner loop, the
compiler prints a value of ( i * z) = ( 1 * 1 = 1);
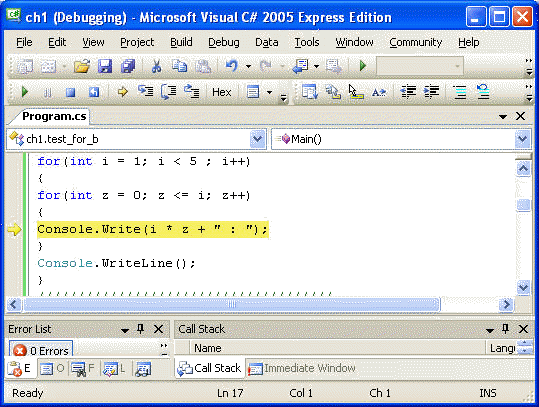
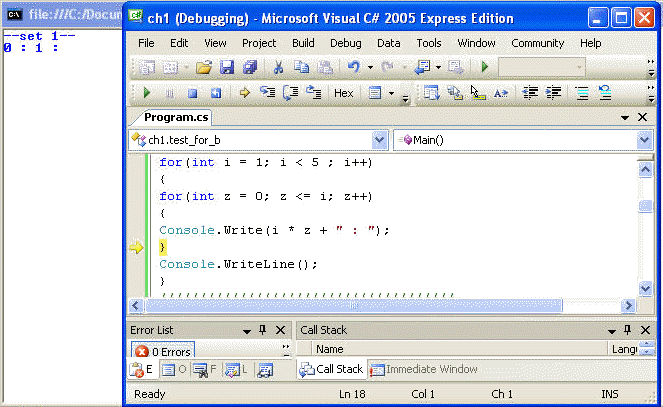
|
Step 6:
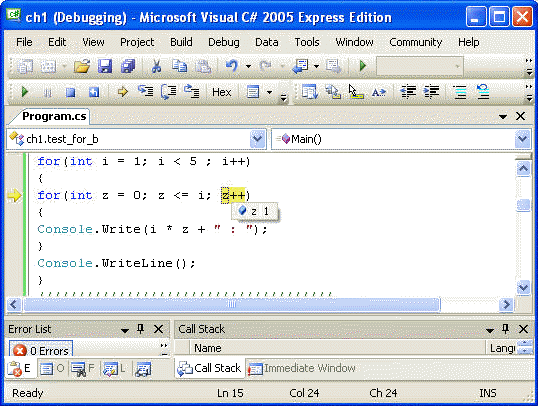
However, this round the value of z increases ( z = 2) exceeds the current
value of i( i=1);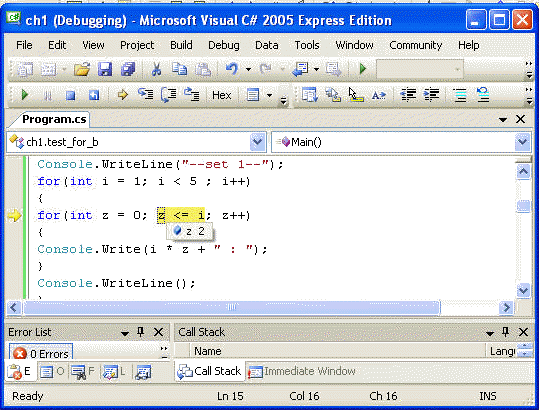
Therefore, as per condition, compiler exits the loop, and
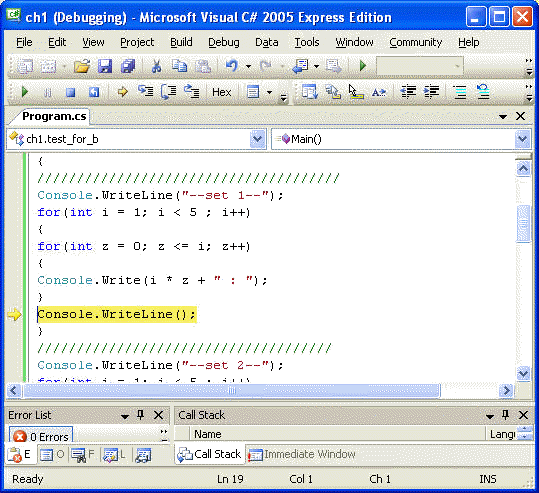
|
Step 7: As we proceed with debugging; the variable i in the outer loop,
increases by 1 ( i =2);
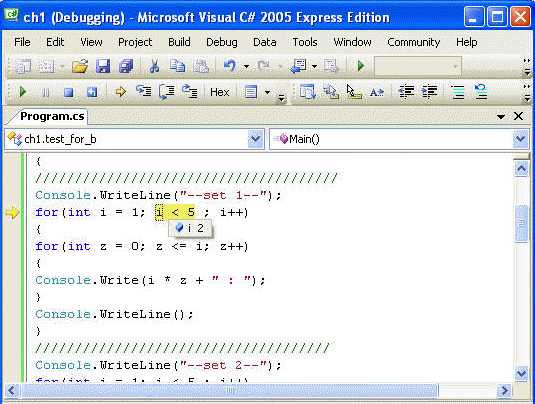
|
Step 8: In the inner loop, the value of z = 2;
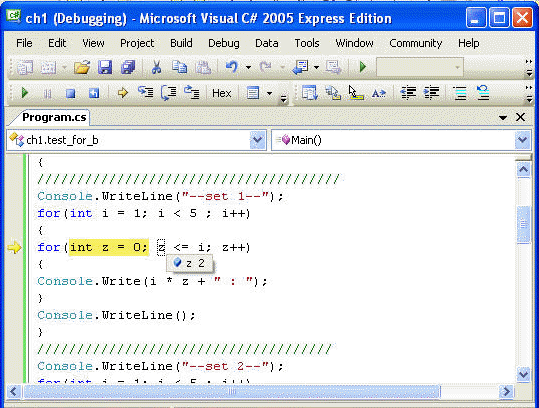
However, as in the loop, the value of z is reset to 0; (where as i = 2,
meaning
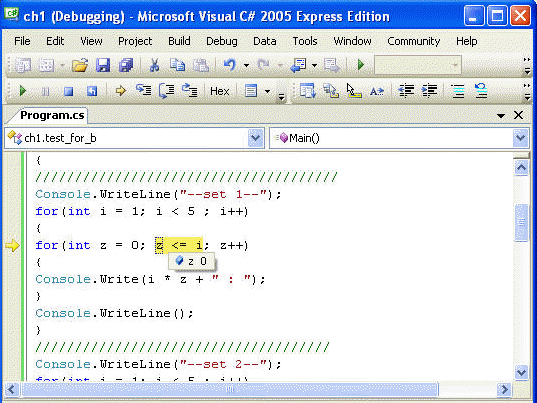
|
Step 9: now note the value of ( i * z)
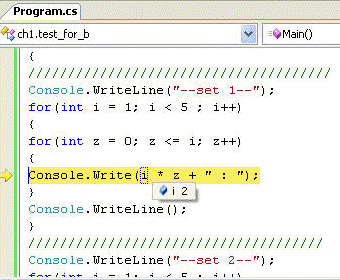 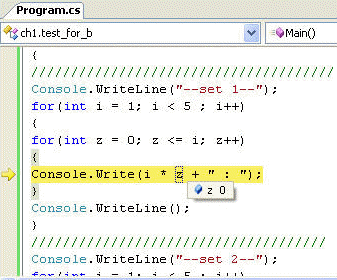
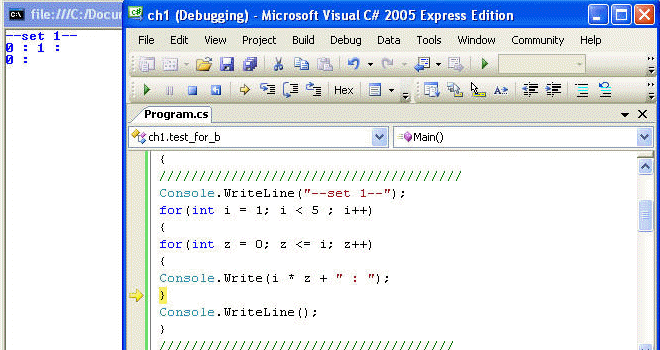
|
Step 8: The routine check on z++,
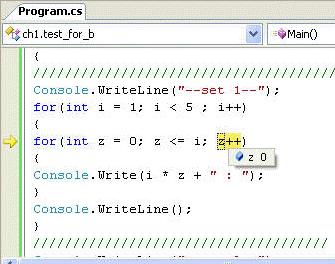 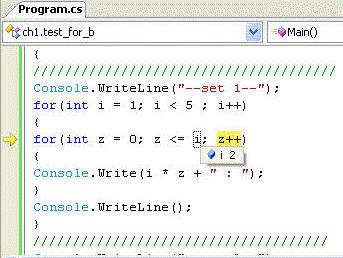
at this point the values of i and z are 2 and 1 respectively;
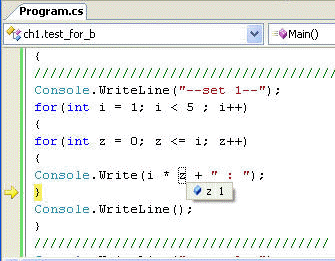 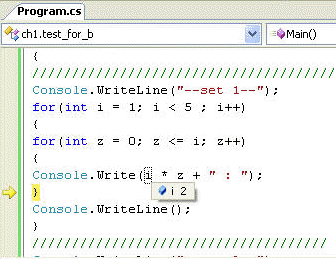
we get the following output
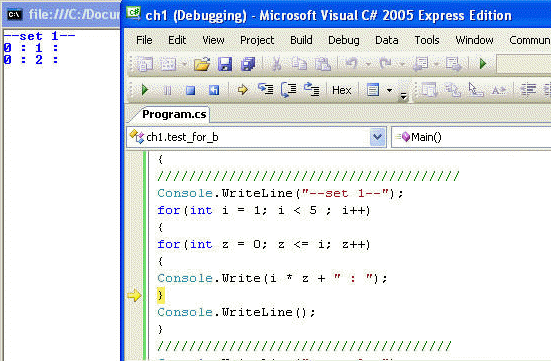 |
|
|
|
|
|
|
|
|
|