|
- A thread is a basic processing unit to which an operating system
allocates processor time, and more than one thread can be executing code
inside a process.
- Every Java program has at least one thread, the thread that executes
the Java program. It is created when you invoke the static main method
of your Java class.
|
Creating Threads :
- Extend the java.lang.Thread class
- Implement the java.lang.Runnable
interface.
|
- Thread object is created using new key word, then invoke "start
"method to activate the thread.
- When a thread is started, its run method is executed.
- When the run method returns or throws an exception, the thread
dies and get cleaned with garbage collection.
- Thread can't be generic
|
Every Thread has a state and a Thread can be in one of the following
six states. |
- new. A state in which a thread has not been started.
- runnable. A state in which a
thread is executing.
- blocked. A state in which a
thread is waiting for a lock to access an object.
- waiting. A state in which a
thread is waiting indefinitely for another thread to perform an
action.
- timed__waiting. A state in
which a thread is waiting for up to a specified period of time for
another thread to perform an action.
- terminated. A state in which a
thread has exited.
|
|
The values that represent these states are encapsulated in the
java.lang.Thread.State enum. The members of this enum are NEW, RUNNABLE,
BLOCKED, WAITING, TIMED__WAITING, and TERMINATED |
|
//import java.lang.reflect.*;
import java.io.*;
import java.net.*; // for datagram
public class Test_this extends Thread {
static public void main( String[] args )throws IOException
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("-->Welcome to thread building<--");
System.out.print("type name ");
String str = br.readLine();
Thread t = Thread.currentThread();
if(t.isAlive())
{
System.out.println( " get name '" + t.getName()+ "' 'id' " + t.getId());
}
thread_this tt = new thread_this();
tt.process_thread(str);
} }
class thread_this extends Thread{
public static void process_thread(String str)
{
Thread t = new thread_this();
t.setName(str);
t.start();
System.out.println( " get name " + t.getName()+ " id " + t.getId());
}
}
|
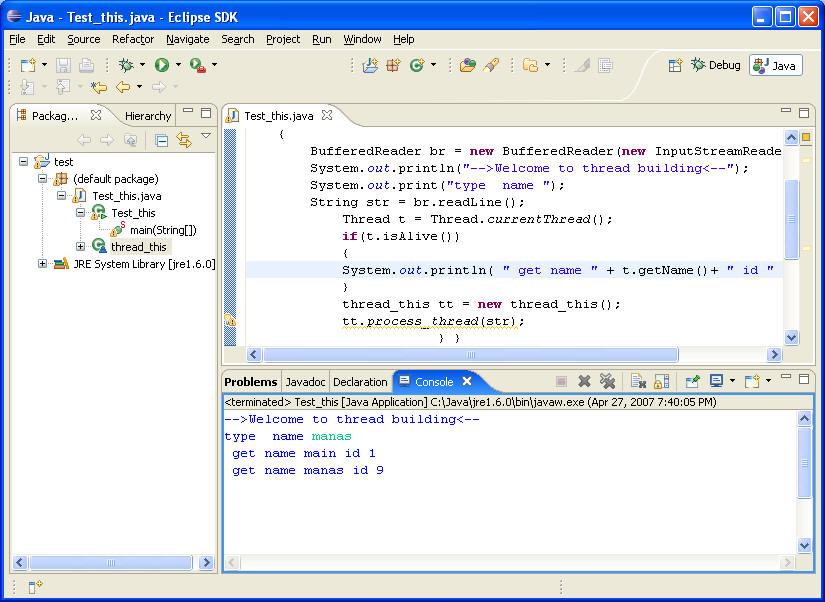 |
Complete Code :
//import java.lang.reflect.*;
import java.io.*;
import java.net.*; // for datagram
public class Test_this extends Thread {
static public void main( String[] args )throws IOException
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("-->Welcome to thread building<--");
System.out.print("type name ");
String str = br.readLine();
Thread t = Thread.currentThread();
if(t.isAlive())
{
System.out.println( " get name " + t.getName()+ " id " + t.getId());
}
System.out.println( " --- " );
thread_this t_this = new thread_this();
//thread_that t_that = new thread_that();
t_this.process_thread(str);
} }
class thread_this extends Thread{
public static void process_thread(String str)
{
Thread t = new thread_this();
t.setName(str);
t.start();
Thread nt;
String s = "";
System.out.println( " get name " + t.getName()+ " id " + t.getId());
System.out.println( "get.State() " + t.getState());
System.out.println( "getPriority " + t.getPriority());
for (int i =1; i < 4; i++)
{
nt = new Thread(s = str+ i);
System.out.println("\tThread : "+ i);
System.out.println( " get name " + nt.getName()+ " id " + nt.getId() + "
state " + t.getState());
}
}
}
|
|
|
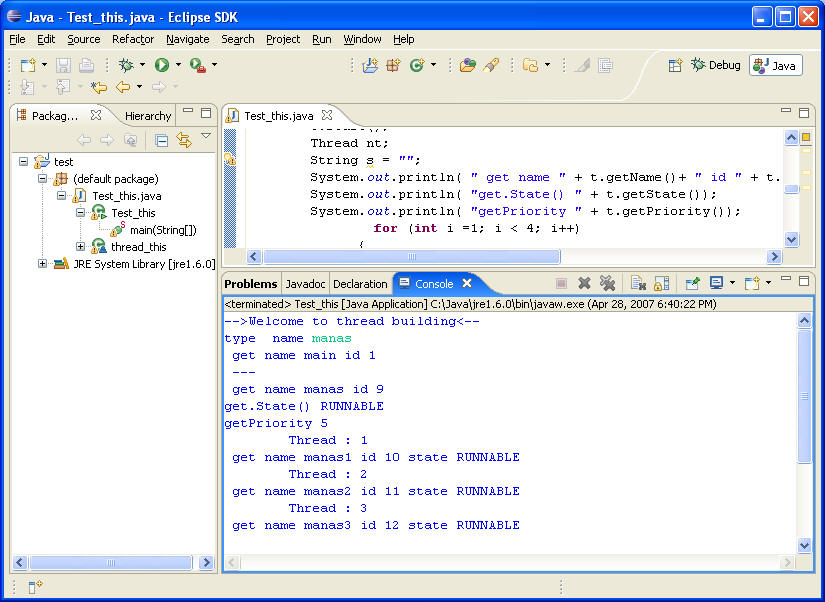 |