The input stream is super class and acts as a super class to all input
classes. |
import java.io.*;
public class Test_input {
public static void main(String[] args)
{
// TODO Auto-generated method stub
System.out.println("Please type something");
byte[] b = new byte[200];
try
{
System.in.read(b);
}
catch(Exception e) { System.out.println("there is an error");}
String str = new String(b);
System.out.println("You Typed\t"+ str);
}
}
|
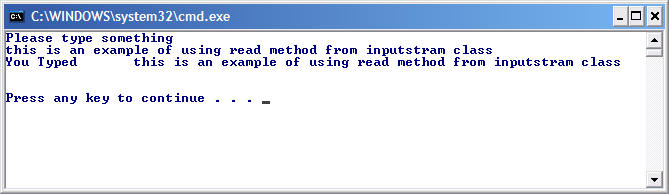
In the above code the byte is set to 200, but below the byte limit is
set to 20, and string is immutable. So it is your choice and decision when
to limit the byte size using an inputstream class.
|
import java.io.*;
public class Test_Input2 {
public static void main(String[] args)
{
// TODO Auto-generated method stub
System.out.println("Please type something");
byte[] b = new byte[20];
try
{
System.in.read(b);
}
catch(Exception e) { System.out.println("there is an error");}
String str = new String(b);
System.out.println("You Typed\t"+ str + " total byte " + (byte)str.length());
}
}
|
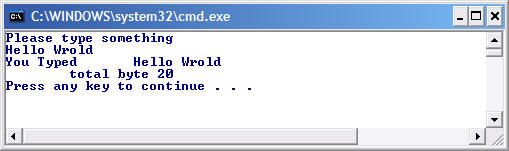
If you exceed the byte limit, inputstream will ignore the rest
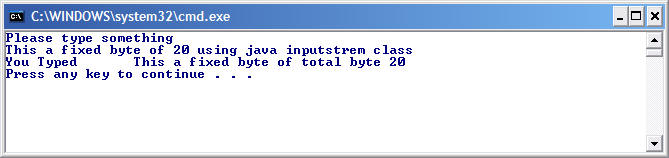
|
java.io
Class InputStream
java.lang.Object
java.io.InputStream
- All Implemented Interfaces:
-
Closeable
- Direct Known Subclasses:
-
AudioInputStream,
ByteArrayInputStream,
FileInputStream,
FilterInputStream,
InputStream,
ObjectInputStream,
PipedInputStream,
SequenceInputStream,
StringBufferInputStream
public abstract class InputStream
extends Object
implements Closeable
This abstract class is the superclass of all classes representing an
input stream of bytes.
Applications that need to define a subclass of InputStream
must always provide a method that returns the next byte of input.
- Since:
- JDK1.0
- See Also:
-
BufferedInputStream ,
ByteArrayInputStream ,
DataInputStream ,
FilterInputStream ,
read() ,
OutputStream ,
PushbackInputStream
Method Summary |
int |
available()
Returns the number of bytes that can be read (or skipped
over) from this input stream without blocking by the next caller of a
method for this input stream. |
void |
close()
Closes this input stream and releases any system resources
associated with the stream. |
void |
mark(int readlimit)
Marks the current position in this input stream. |
boolean |
markSupported()
Tests if this input stream supports the mark
and reset methods. |
abstract int |
read()
Reads the next byte of data from the input stream. |
int |
read(byte[] b)
Reads some number of bytes from the input stream and stores
them into the buffer array b . |
int |
read(byte[] b, int off, int len)
Reads up to len bytes of data from the input
stream into an array of bytes. |
void |
reset()
Repositions this stream to the position at the time the
mark method was last called on this input stream. |
long |
skip(long n)
Skips over and discards n bytes of data from
this input stream. |
|
|
|