|
A switch works with the byte , short ,
char , and int primitive data types. It also works
with enumerated types (discussed in
Classes and Inheritance) and a few special classes that "wrap" certain
primitive types:
Character ,
Byte ,
Short , and
Integer (discussed in
Simple Data Objects ). |
import java.io.*;
//javac control_switchcase.java
//throws exception is needed to cat error with try/case
public class control_switchcase
{
public static void main(String args[])throws IOException
{
//implementation with code satrs
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
String str= "";
////////////////
try {
System.out.print("enter first number : ");
str = br.readLine();
int n = Integer.parseInt(str);
switch (n)
{
//case 0: System.out.println(" You entered " + n);
//break;
case 1: System.out.println(" You entered one " + n);
break;
case 2: System.out.println(" You entered two " + n);
break;
case 3: System.out.println(" You entered three " + n);
break;
case 4: System.out.println(" You entered " + n);
break;
case 5: System.out.println(" You entered " + n);
break;
default:System.out.println(" You entered " + n + " out of range");
break;
}
System.out.println(" Thanks for visitng " + n);
////////////////
System.exit(0); //otherwise cursor will not return to DOS
//
}//try block
catch(NumberFormatException e) { System.out.println("data was blank");}
///
System.out.println();
}
}
|
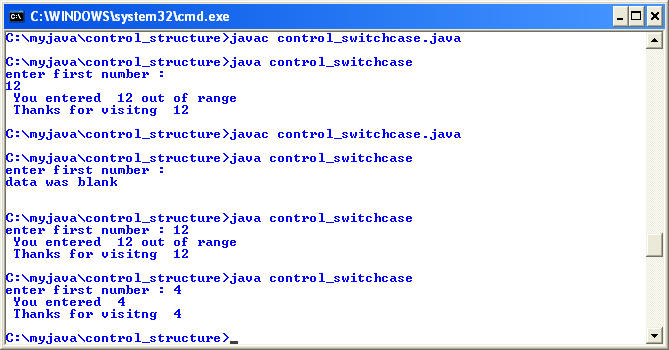 |
With Eclipse |
import java.util.*;
import java.io.*;
//javac clas_name.java
public class test
{
private static int player_no = 24;
public static String str;
public test()
{ System.out.println("This is a constructor with no param");
}
public static void main(String[] args) throws IOException
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Type a line to end type exit at the end :");
try {
System.out.print("enter first number : ");
str = br.readLine();
int n = Integer.parseInt(str);
switch (n)
{
// case 0: System.out.println(" You entered " + n);
// break;
case 1: System.out.println(" You entered :"+ n +" Freshman " );
break;
case 2: System.out.println(" You entered : " + n+ "Sophomore");
break;
case 3: System.out.println(" You entered : " + n + "Junior ");
break;
case 4: System.out.println(" You entered : " + n + "Senior ");
break;
default:System.out.println(" You entered " + n + " out of range");
break;
}
System.out.println(" Thanks for visitng " + n);
////////////// //
System.exit(0); //otherwise cursor will not return to DOS
//
}//try block
catch(NumberFormatException e) { System.out.println("data was blank");}
// /
System.out.println();
}
}
|
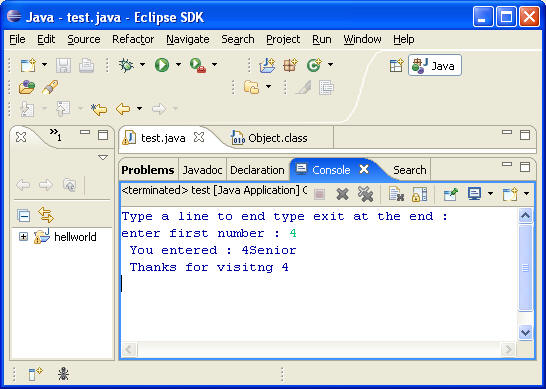 |