This example introduces scope of a class and variables; also shows
encapsulation.
- private and public fields.
- class constructor
- Scanner sc = new Scanner(System.in);
- nextLine();
|
Encapsulation is a property that allows of an object or filed
to put a constraints to the external classes; The private variables
can only be seen or modified by use of object
accessors and mutators methods. The
mutator methods are void type, do not return any value to the callers. |
Now when it comes to using accessors, consider the example shown below,
the public class scope_client has the entry point of this program (main),
compiler will create two classes like "scope_client.class" and "scope_server.class";
but the signature to run this code must have the name like "scope_client.java".
|
import java.io.*;
import java.util.*;
// javac scope_client.java
public class scope_client
{
public static void main(String args[])throws IOException
{
System.out.println("Client Starts");
//creating object where ss is reference to the class simple server_1
scope_server ss = new scope_server();
ss.process();
System.out.println("Called a public variable: " + ss.str);
System.out.println("Client ends");
}//end of main
}//end of class
class scope_server
{
//constructor
public scope_server(){ System.out.println("Server Constructor Starts as an
object is created");}
//these are the fileds
private int n1 = 0;
public String str="";
//method starts
//accesor type public
//return type void
//() place holder for parameters
public void process() throws IOException
{
Scanner sc = new Scanner(System.in);
System.out.print("Please type your name :");
str = sc.nextLine();
System.out.print("Please type age:");
n1 = sc.nextInt();
str += " : " + n1;
System.out.println();
}//end of process
}//end of class
|
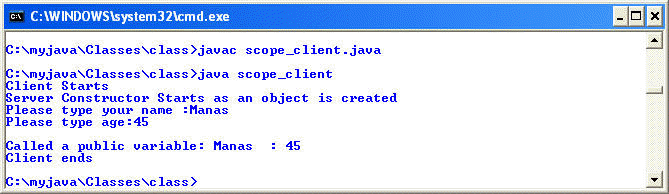 |
|
|
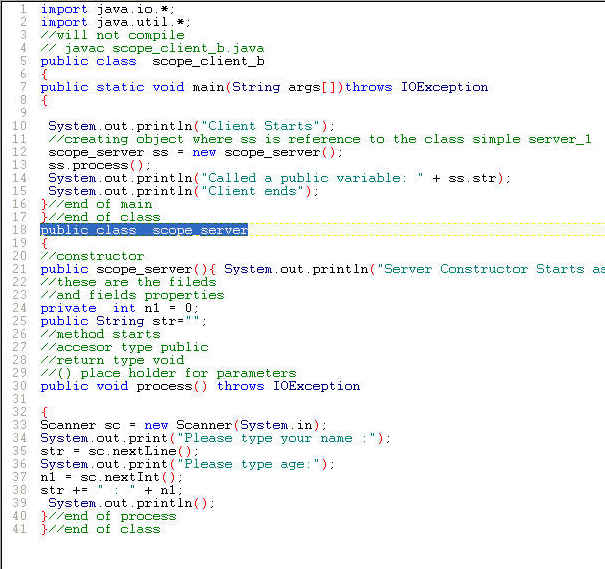 |
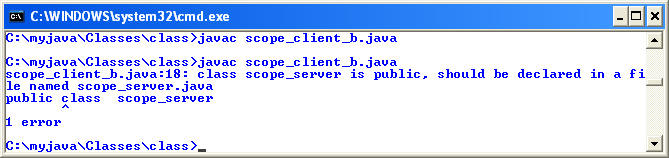 |
I noticed one glitch with nextInt or line, it does not exit after
keeping either of the variables empty., as show n in the picture below.
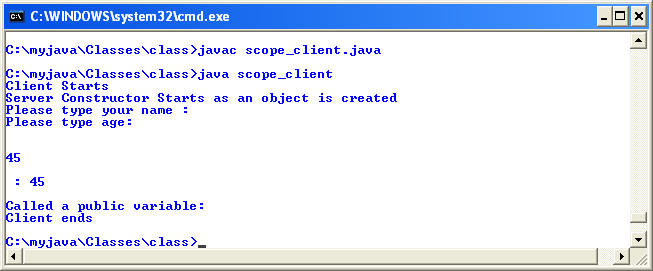 |
|