abstract_class_method.htm |
Declaring a class an abstract enforces the following rules.
- an abstract method can only remain in an interface or abstract class
- an abstract base class must contain an abstract method.
- extended class must create the instance of the abstract method or
methods.
- While creating an instance of the abstract method that was enforced by
the abstract class, the method or methods in the extended class would not
be declared with abstract key word.
- You can't create an instance of an Abstract class.
- abstract class is similar to virtual class of C++ and C#.
|
|
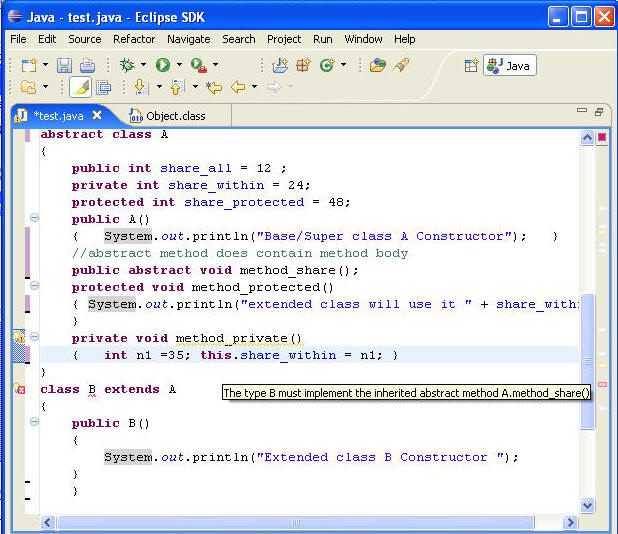 |
Consider the following codes, after editing the preceding one, the
function "method-share" in class B now contains a body that would perform
some work. And note that there is no error sign as seen before creating the
instance of an abstract method present in the base class.
abstract class A
{
public int share_all = 12 ;
private int share_within = 24;
protected int share_protected = 48;
public A()
{ System.out.println("Base/Super class A Constructor"); }
//abstract method does contain method body
public abstract void method_share();
protected void method_protected()
{ System.out.println("extended class will use it " + share_within);
}
private void method_private()
{ int n1 =35; this.share_within = n1; }
}
class B extends A
{
public B()
{ System.out.println("Extended class B Constructor "); }
public void method_share()
{
System.out.println("class B inherited " +
"abstract class from A "); }
}
|
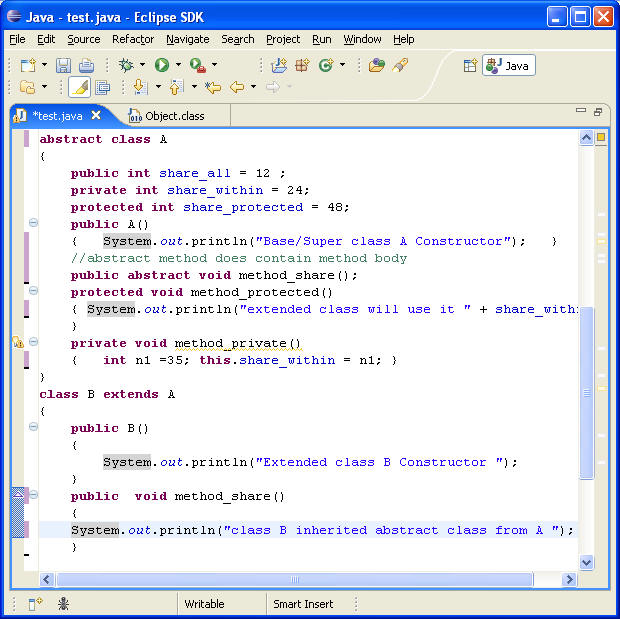 |
Complete code:
import java.io.*;
import java.io.IOException;
//javac clas_name.java
public class test
{
String str = "Helloworld";
public static void main(String[] args) throws IOException
{
InputStreamReader isr = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(isr);
// needs throws IOException
System.out.print("Type some thing : ");
String str =br.readLine();
B b = new B();
System.out.println("---------------");
System.out.println("Reading integers from Base via derived class");
System.out.println("The value of share_all integer :"+ b.share_all);
System.out.println("The value of share_protected from Base via derived
class :"+ b.share_protected);
System.out.println("---------------");
System.out.println("calling methods from Base via derived class");
b.method_protected();
b.method_share();
}
}
abstract class A
{
public int share_all = 12 ;
private int share_within = 24;
protected int share_protected = 48;
public A()
{ System.out.println("Base/Super class A Constructor"); }
//abstract method does contain method body
public abstract void method_share();
protected void method_protected()
{
System.out.println("\toriginal value of share_within : " + share_within);
method_private();
System.out.println("\tchanged value of share_within : " + share_within);
}
private void method_private()
{ int n1 =35; this.share_within = n1; }
}
class B extends A
{
public B()
{ System.out.println("Extended class B Constructor "); }
//instance of class A's abstract method,
method_share
public void method_share()
{
share_protected += 10;
System.out.println("class B inherited " +
"abstract class from A " + share_protected); }
}
|
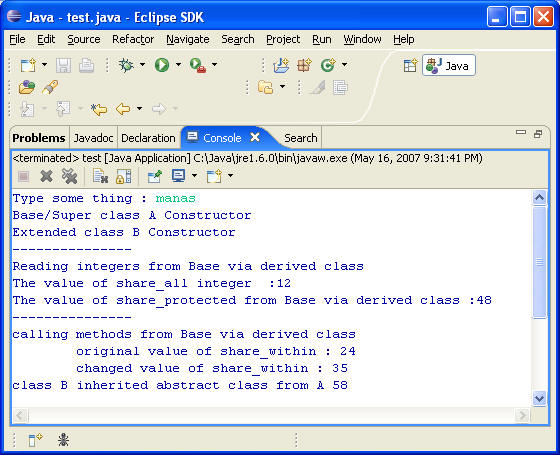 |
|
|
|