Example of using FileFilter, also See
FilenameFilter |
listFiles
public File[] listFiles(FileFilter filter)
- Returns an array of abstract pathnames denoting the files
and directories in the directory denoted by this abstract
pathname that satisfy the specified filter. The behavior of this
method is the same as that of the
listFiles() method, except that the pathnames in the
returned array must satisfy the filter. If the given
filter is null then all pathnames are
accepted. Otherwise, a pathname satisfies the filter if and only
if the value true results when the
FilenameFilter.accept(java.io.File, java.lang.String)
method of the filter is invoked on the pathname.
-
- Parameters:
filter - A filename filter
- Returns:
- An array of abstract pathnames denoting the files and
directories in the directory denoted by this abstract
pathname. The array will be empty if the directory is empty.
Returns
null if this abstract pathname does not
denote a directory, or if an I/O error occurs.
|
import java.io.File;
import java.io.FileFilter;
//javac Test_FileFilter.java
//java -classpath . Test_FileFilter
public class Test_FileFilter {
public static void main(String[] args) {
// TODO Auto-generated method stub
File myDir = new File("c:/myjava");
GetFileFilter select = new GetFileFilter(myDir);
File[] contents = myDir.listFiles(select);
for (File file : contents) {
System.out.println(file + " is a " + (file.isDirectory() ?
"directory" : "file"));
}
}
}
class GetFileFilter implements FileFilter
{
private String dir;
private String fileExtension;
//the constructor that will receive directory and file extension
public GetFileFilter( File dir)
{
this.dir= fileExtension;
System.out.println("costructor gets \t" + "dir : " +dir );
}
//A class which implements the FilenameFilter interface
//must define this method
public boolean accept(File dir) {
boolean fileOK = true;
if (dir != null) {
fileOK = dir.getName().endsWith(".java");
return fileOK;
}
else { return (false); }
}
}
// end of getFilter
|
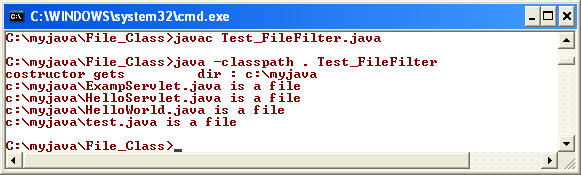
|
|