There is some rule of assignments while handling variables
- Implicit casting or conversion
- higher-type ht = lower type; // implicit casting allowed
- double d ;
- int i ;
- d = i ; // implicit casting allowed
- lower-type lt = (lower-type) ht; // explicit
casting allowed
- one-type ot = (one-type)anoather-type;//explicit conversion
- you are not allowed to assign
- lower- type lt = higher type type // implicit casting allowed
|
The conversions (from a smaller class or data type to a larger) can
be implicit, but it's good practice to convert explicitly. The
conversions from larger to smaller data types must be explicitly
converted, or cast. |
import java.io.*;
//this won't compile
// javac double_or_int.java
public class double_or_int
{
public static void main(String args[])throws IOException
{
/*this is a comment
compile as
javac multi_comment.java
call as java simple_comment */
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Type 1st double number only: ");
String str = "";
str = br.readLine();
System.out.println(str);
double d1 = Double.parseDouble(str);
//System.out.println();
System.out.print("Type 1st integer number only: ");
str = br.readLine();
System.out.println(str);
int n1 = Integer.parseInt(str);
System.out.println("Added up n1 + n1 = " + (d1+n1));
System.out.println("Divide up d1 / n1 = " + (d1 /n1));
double d2 = n1 /n1 + d1;
System.out.println("Divide up double d2= n1 /n1 + d1 = " + d2);
int n3 = n1 /n1 + d1;
System.out.println("Divide up int n3 = n1 /n1 + d1 = " + n3);
}
}
|
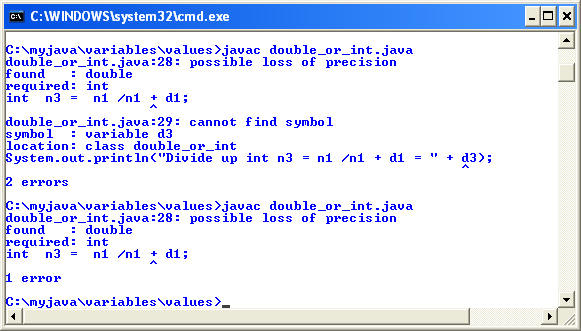 |
The following code will compile, but result will surprise you.
import java.io.*;
//this won't compile
// javac double_or_int_b.java
public class double_or_int_b
{
public static void main(String args[])throws IOException
{
/*this is a comment
compile as
javac multi_comment.java
call as java simple_comment */
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Type 1st double number only: ");
String str = "";
str = br.readLine();
System.out.println(str);
double d1 = Double.parseDouble(str);
//System.out.println();
System.out.print("Type 1st integer number only: ");
str = br.readLine();
System.out.println(str);
int n1 = Integer.parseInt(str);
System.out.println("Added up n1 + n1 = " + (d1+n1));
System.out.println("Divide up d1 / n1 = " + (d1 /n1));
double d2 = n1 /n1 + d1;
System.out.println("Divide up double d2= n1 /n1 + d1 = " + d2);
//now this inludes an inplicit casting
//higher type = accepting lower type or eqaual
double n3 = n1 /n1 + d1;
System.out.println("Divide up double n3 = n1 /n1 + d1 = " + n3);
}
}
|
If you wanted to have n1 should be divide by n1 + d1; the grammar
precedence rule was incorrect. |
import java.io.*;
//this won't compile
// javac double_or_int_c.java
public class double_or_int_c
{
public static void main(String args[])throws IOException
{
/*this is a comment
compile as
javac multi_comment.java
call as java simple_comment */
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Type 1st double number only: ");
String str = "";
str = br.readLine();
System.out.println(str);
double d1 = Double.parseDouble(str);
//System.out.println();
System.out.print("Type 1st integer number only: ");
str = br.readLine();
System.out.println(str);
int n1 = Integer.parseInt(str);
System.out.println("Added up n1 + n1 = " + (d1+n1));
System.out.println("Divide up d1 / n1 = " + (d1 /n1));
double d2 = n1 /n1 + d1;
System.out.println("Divide up double d2= n1 /n1 + d1 = " + d2);
//now this inludes an inplicit casting
//higher type = accepting lower type
double n3 = n1 /(n1 + d1);
System.out.println("Divide up double n3 = n1 /(n1 + d1) = " + n3);
}
}
|
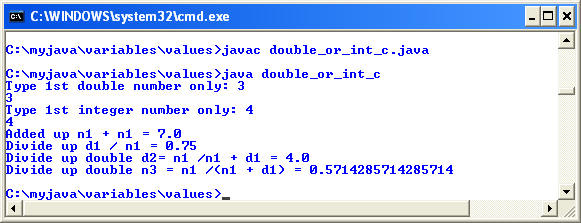 |
import java.io.*;
// javac double_or_int_d.java
public class double_or_int_d
{
public static void main(String args[])throws IOException
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String str = "";
System.out.print("Type 1st double number only: ");
try
{
str = br.readLine();
if(str!=""){
System.out.println(str);
double d1 = Double.parseDouble(str);
//implicit conversion is not allowed
//int n1 = d1;
int n1 = (int)d1;
System.out.println("\texplicit conversion \nfrom double to integer : " +
n1 + " is an integer type");
}
}
catch(NumberFormatException e) { System.out.println("data was blank");}
//implicit conversion from integer to double
System.out.print("Type an integer number only: ");
try
{
str = br.readLine();
if(str!=""){
double d1 = Double.parseDouble(str);
int n2 = Integer.parseInt(str);
d1 = n2;
System.out.println("\timplicit conversion from \ninteger to double : " +
d1 + " is an double type ");
}
}
catch(NumberFormatException e) { System.out.println("data was blank");}
}
}
|
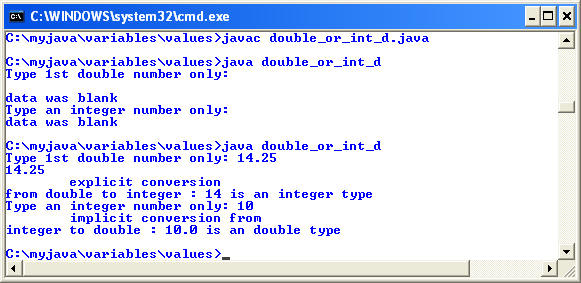 |
|