This example show how to read object from a file using the
followings.
- ObjectOutputStream(xyz)
- FileOutputStream(abc);
- ObjectInputStream
- readObject()
|
It is worth of revising OutputStreamWriter and
InputStreamReader
|
Algorithms of this example is shown below.
- An instance of FileOutputStream allows the bytes to be
written to file that will be created on the fly, using File
class.
- The object created with FileOutputStream, is then presented
to the contstructor of the class ObjectOutputStream, which
allows the objects to be written in the file .
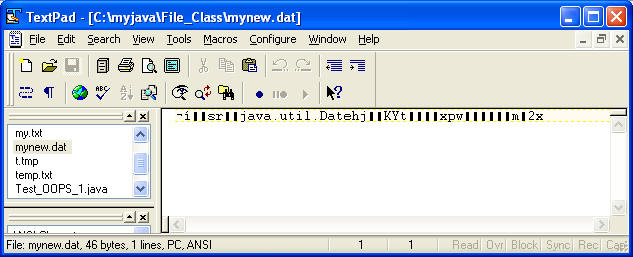
- As we know these will appear as text garbage to in a text
reader, unless we read via input stream readers as we have done
below.
|
import java.io.*;
import java.io.LineNumberReader;
import java.util.*;
//javac Test_OOPS_WR.java
//java -classpath . Test_OOPS_WR
public class Test_OOPS_WR{
/**
* @param args throws exception needed with LineNumberReader
*/
public static void main(String[] args) throws Exception {
// TODO Auto-generated method stub
File myFile = new File("c:/myjava/File_Class/mynew.dat");
Date today = new Date();
try {
FileOutputStream fos = new FileOutputStream(myFile);
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(today);
oos.close();
System.out.println("doing good");
} catch (IOException e) {
System.out.println(e.toString());
}
System.out.println("Written to the file : " );
OOPS_Read oops = new OOPS_Read();
oops.method_objRead(today);
}
}
class OOPS_Read
{
Date today = null;
public void method_objRead(Date today)
{
File f = new File("c:/myjava/File_Class/my.dat");
ObjectInputStream in = null;
try {
in = new ObjectInputStream(new FileInputStream(f));
this.today = (Date)in.readObject();
in.close();
}
catch(Exception e)
{
e.printStackTrace();
}
System.out.println("Readings are : " + today );
}
}
|
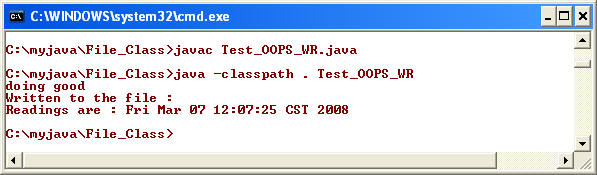 |