In this example we are learning the followings.
- Creating an instance of a class
- Creating an instance of a method.
- declaring a variable and then initializing a variable.
- returning value by a method.
|
import java.util.*;
import java.io.*;
//javac test_operator.java
public class test
{
//declaring variable
private int player_no;
public test(){ System.out.println("This is a constructor with no param");}
public static void main(String[] args) throws IOException
{
// creating instance and object "t" of the test class
test t = new test();
System.out.println("Player no : " +t.get_no());
}
private int get_no()
{
System.out.println("This method returns value to the calling function : "
);
//intializing variable playr_no
player_no = 12;
return player_no;
}
}
|
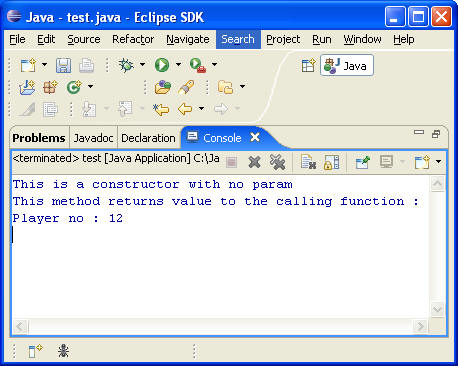
|
using command line
import java.util.*;
import java.io.*;
//javac test_instance.java
public class test_instance
{
//declaring variable
private int player_no;
public test_instance (){ System.out.println("This is a constructor with no
param");}
public static void main(String[] args) throws IOException
{
// creating instance and object "t" of the test class
test_instance t = new test_instance();
System.out.println("Player no : " +t.get_no());
}
private int get_no()
{
System.out.println("This method returns value to the calling function : "
);
//intializing variable playr_no
player_no = 12;
return player_no;
}
}
|
This example was created with text-pad trial version.
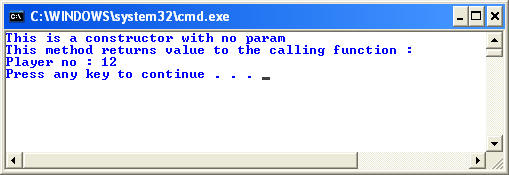 |