Everything is class in java.
- If you are using some tool like Eclipse or Net Bean; your
application will be layered within a project/package, and irrespective
of tools, there would be a main controller or entry point in your code.
- How it is architected?
- Class
- fields to all methods in the class
- public constructor
- methods or function
- public static void or return main (arguments ) condition like
throws IOException
OR
- public static void or return main (arguments ) condition like
throws
- fields to the local method.
|
declaration a class
- ["public"] ["abstract"|"final"]"class" class_name ["extends"
object_name]
"{"
// properties declarations
// behavior declarations
}
|
field declaration
-
Scope of the field : [ "public" | "private" | "protected" ] [ "final" ]
[ "static" | "transient" | "volatile" ]
-
data type : int, Sting, double , long , float
-
declaration only : scope data_type field_name
-
initialization of declared field field_name = value;
-
declaration and initialization : data_type var_name = value
;
|
By convention, the first letter should be capitalized.
This type of naming convention is known as Pascal naming convention
or Upper Camel Case. According to the lower camel naming convention, initial
letter is in small cap.
Method and field names use the lower camel naming convention.
|
|
Command Line code
import java io.*;
//javac simple.java
public class simple
{
public static void main(String args[])
{
System.out.println("Hell World");
}
}
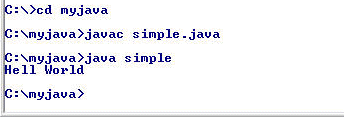
|
Using GUI- Swing
import javax.swing.*;
public class test {
public static void main(String[] args) {
String str = "Hello Wrold";
JOptionPane.showMessageDialog(null, str) ;
}
}
|
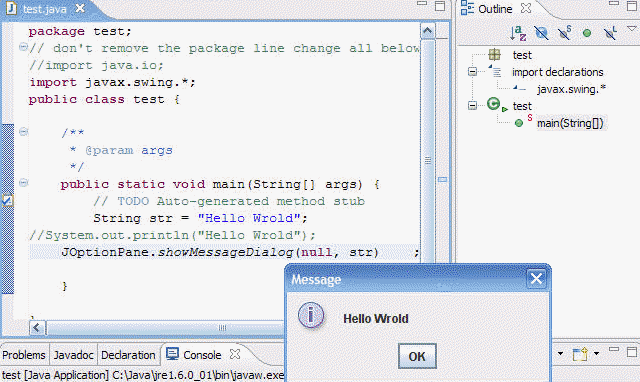 |
|
|