comparing Map with TreeMap |
- public class TreeMap
- extends
AbstractMap
- implements
SortedMap,
Cloneable,
Serializable
Red-Black tree based implementation of the SortedMap interface.
This class guarantees that the map will be in ascending key order, sorted
according to the natural order for the key's class (see
Comparable), or by the comparator provided at creation time, depending
on which constructor is used.
|
Map has the following feature
- No duplicate Data in any case.
- The condition below shows that first string has duplicate Dave, the
first one is overridden ( should have been Dave=Ziba, but it would be
Dave=Jones) and the sequence in the leading(first) data index set the
index for the second one.
- Also View TreeSet_Iterator_Generics1 to compare the uses of TreeSet/TreeMap
|
Example
import java.io.*;
import java.util.*;// Arrays.sort
public class Test_This {
public static void main(String[] args) throws IOException{
// TODO Auto-generated method stub
Process pp = new Process();
pp.method_1();
}
}
class Process
{
String first[] = {"First Name","Andrew ","James","Cathy","Peter","Dave",
"Dave"};
String[] second = {"Last Name","Smith","Cole","Leghorn","Garet","Ziva",
"Jones"};
public Process(){System.out.println("Default) constructor");}
public void method_1()
{
TreeMap tm = new TreeMap();
for(int i = 0; i < first.length; i++)
{
//map.put(arg0, arg1)
tm.put((first[i]),(second[i]));
}
System.out.println(tm);
Map map = new HashMap();
for(int i = 0; i < first.length; i++)
{
map.put((first[i]),(second[i]));
}
System.out.println(map);
}
}
|
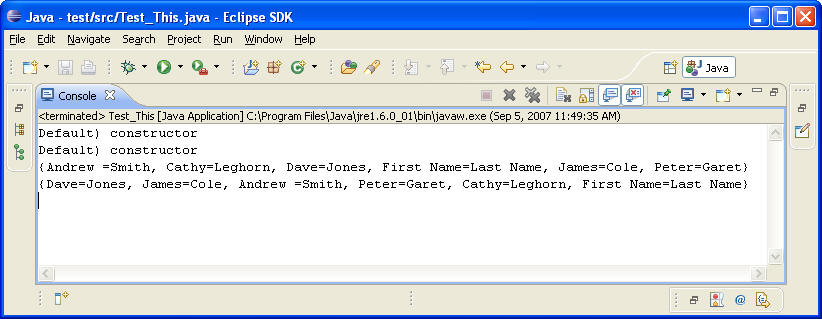 |
Example 2
import java.io.*;
import java.util.*;// Arrays.sort
public class Test_This {
public static void main(String[] args) throws IOException{
Process pp = new Process();
pp.method_1();
}
}
class Process
{
String[] first = {"A","B","D","C","E","F", "F"};
String[] second = {"1","2","3","4","5","6", "5"};
public Process(){System.out.println("Default) constructor");}
public void method_1()
{
TreeMap tm = new TreeMap();
System.out.println("TreeMap map= TreeMap");
for(int i = 0; i < first.length; i++)
{
tm.put((first[i]),(second[i]));
}
System.out.println(tm);
System.out.println("SortedMap map= TreeMap");
SortedMap smp = new TreeMap();
for(int i = 0; i < first.length; i++)
{
//map.put(arg0, arg1)
smp.put((first[i]),(second[i]));
}
System.out.println(smp);
System.out.println("Map mp= Hastable");
Map map = new Hashtable();
for(int i = 0; i < second.length; i++)
{
//map.put(arg0, arg1)
map.put((first[i]),(second[i]));
}
System.out.println(map);
System.out.println("Map hasmp= new HashMap");
Map hasmap = new HashMap();
for(int i = 0; i < second.length; i++)
{
hasmap.put((first[i]),(second[i]));
}
System.out.println(hasmap);
}
}
|
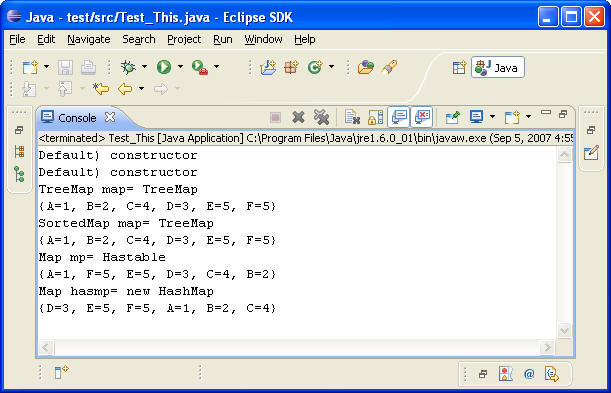 |
|
|
|