Map_HashMap_MapEntry_iteration.htm |
Objectives :
- Map map = new HashMap()
- Set set = map.entrySet();
- Iterator it = set.iterator();
- map.put(arg0, arg1)
- Arrays to Map.put with for loop
- Map.Entry(), getKey(), getValue
|
A Map is a storage that hold key to value mappings.
- both inserting and searching data are quick.
- HashMap is unsynchronized and Hashtable is synchronized.
- HashMap is the faster than Hashtable.
- HashMap allows null where Hashtable does not allow null.
- If you need to maintain map keys in an ordered fashion, you should
use TreeMap.
- Map has get and put, where as Set does not
|
* The Collection interface is a group of objects, with
duplicates allowed.
* The Set interface extends Collection but won't allow
duplicate values.
* The List interface extends Collection, allows duplicates, and
introduces positional
indexing.
* The Map interface extends neither Set nor Collection.
|
Class |
Description |
AbstractMap |
is a super Class, implements of the Map Interface
|
HashMap |
extends AbstractMap to use hashTable |
TreeMap |
extends Abstractmap to use Tree |
|
|
|
|
|
|
|
The Map classes
constructors
HashMap() default
HashMap(Map m) takes map object
HashMap(int capacity) size
HashMap(int capacity, float fill)
---------------Methods----------
boolean equals(Object obj) returns true
when object equals
to Map.Entry key
Object getKey
Object getValue
int hashCode
Object seValue(Object obj) sets this map.
Entry to object value.
|
Example
import java.io.*;
import java.util.List;
import java.util.Arrays;// to use arrays
import java.util.*;// Arrays.sort
import java.util.Enumeration;
public class Test_This {
public static void main(String[] args) throws IOException{
// TODO Auto-generated method stub
Process p1 = new Process();
p1.method_1();
}
}
class Process
{
String first[] = {"Java","A+","C#","XML","JSP","PHP","Z", "C#"};
public Process()
{
System.out.println("Default) constructor");
}
public void method_1()
{
Map map = new HashMap();
for(int i = 0; i < first.length; i++)
{
//map.put(arg0, arg1)
map.put(("key " + i), first[i]);
}
System.out.println(map);
Set set = map.entrySet();
Iterator it = set.iterator();
System.out.println("-----Using Iteration----");
while (it.hasNext())
{
Map.Entry me = (Map.Entry)it.next();
System.out.print(me.getKey()+ " : ");
System.out.print(me.getValue());
System.out.println();
}
}
}
|
Note that HashMap does not sort like TreeMap, nor checks duplicate
values.
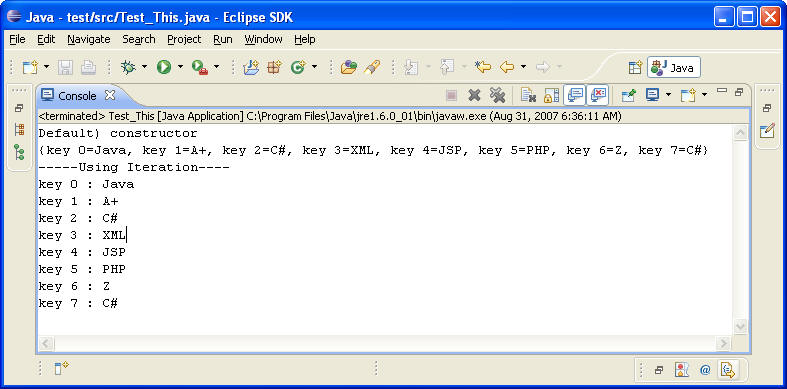
|
|
|
|