Pointer in Unsafe |
Declaring a pointer
int* p1, p2, p3; // Ok
int *p1, *p2, *p3; // Invalid in C#
|
Example |
Description (This table is from msdn2 ) |
int* p |
p is a pointer to an integer |
int** p |
p is a pointer to pointer to an integer |
int*[] p |
p is a single-dimensional array of pointers to integers |
char* p |
p is a pointer to a char |
void* p |
p is a pointer to an unknown type |
Operator/Statement |
Use |
* |
to perform pointer indirection. |
-> |
to access a member of a struct through a pointer. |
[] |
to index a pointer. |
& |
to obtain the address of a variable. |
++ and -- |
to increment and decrement pointers. |
+ and - |
to perform pointer arithmetic. |
==, !=, <, >, <=, and >= |
to compare pointers. |
stackalloc |
to allocate memory on the stack. |
fixed statement |
to temporarily fix a variable in order that its address may be
found. |
|
Example
using System;
using System.Collections.Generic;
//csc unsafe/ unsafe_pointer.cs
namespace Test_This
{
class MainClass
{
public static void Main(string[] args)
{
Console.WriteLine("Hello World!");
//Process pp = new Process();
Process.method_1();
}
}
class Process
{
unsafe public static void method_1()
{
int count = 99;
int* p; // create an int pointer
p = &count; // put address of count into p
Console.WriteLine("Initial value of count is " + *p);
*p = 10; // assign 10 to count via p
Console.WriteLine("New value of count is " + *p);
}
}
}
|
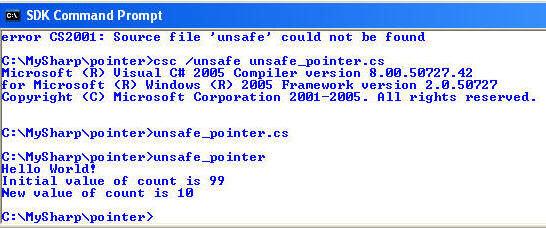 |
|