|
using System;
using System.Threading;
using System.Diagnostics;
// csc thread_delegation_priority_1.cs
//also imples to ThreadStart examples
public class Test
{
static void Main()
{
thread_count cycle = new thread_count();
///set up for count 1
ThreadStart job1 = new ThreadStart(cycle.Count1);
Thread thread1 = new Thread(job1);
thread1.Name ="Count 1 ";
//set up for count 2
ThreadStart job2 = new ThreadStart(cycle.Count2);
Thread thread2 = new Thread(job2);
thread2.Name ="Count 2 ";
//
thread2.Priority = ThreadPriority.Highest;
thread1.Priority = ThreadPriority.Lowest;
thread1.Start();
thread2.Start();
//
Console.ReadLine();
}
}
public class thread_count
{
public void Count1()
{
Console.WriteLine ("Thread name:- {0}",Thread.CurrentThread.Name.ToString());
for (int i=0; i < 10; i++)
{
Console.WriteLine ("Less Priority thread: {0}", i);
//allow other threads to work with sleep
Thread.Sleep(500);
}
}
public void Count2()
{
Console.WriteLine ("\t\t\tThread name: {0}",Thread.CurrentThread.Name.ToString());
for (int i=0; i < 10; i++)
{
Console.WriteLine ("\t\t\tHight Priority thread: {0}", i);
Thread.Sleep(500);
}
// I was curious to know if a process has more work load !
//chek it out, and
//Process newProc = Process.Start("iexplore.exe");
//Process newProc = Process.Start("notepad.exe");
//Console.WriteLine("\t\tNew process started...Must close the application
you open with");
//newProc.WaitForExit();
//newProc.Close();
}
}
|
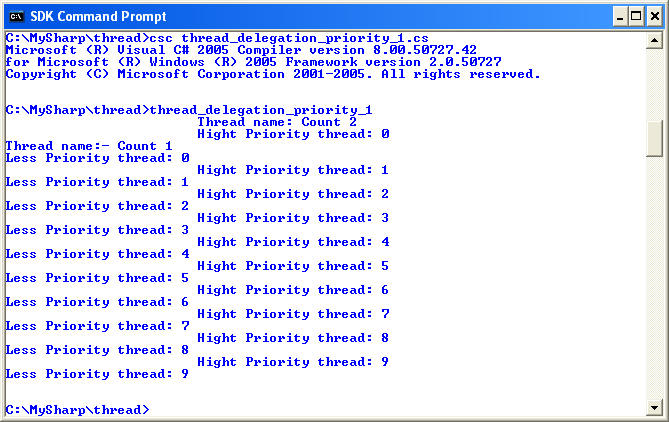 |
|
|
using System;
using System.Threading;
using System.Diagnostics;
// csc thread_delegation_priority_2.cs
//also imples to ThreadStart examples
public class Test
{
static void Main()
{
thread_count cycle = new thread_count();
///set up for count 1
ThreadStart job1 = new ThreadStart(cycle.Count1);
Thread thread1 = new Thread(job1);
thread1.Name ="Count 1 ";
//set up for count 2
ThreadStart job2 = new ThreadStart(cycle.Count2);
Thread thread2 = new Thread(job2);
thread2.Name ="Count 2 ";
//
thread2.Priority = ThreadPriority.Highest;
thread1.Priority = ThreadPriority.Lowest;
thread1.Start();
thread2.Start();
//
Console.ReadLine();
}
}
public class thread_count
{
public void Count1()
{
Console.WriteLine ("Thread name:- {0}",Thread.CurrentThread.Name.ToString());
for (int i=0; i < 10; i++)
{
Console.WriteLine ("Less Priority thread: {0}", i);
//allow other threads to work with sleep
Thread.Sleep(500);
}
}
public void Count2()
{
Console.WriteLine ("\t\t\tThread name: {0}",Thread.CurrentThread.Name.ToString());
for (int i=0; i < 10; i++)
{
Console.WriteLine ("\t\t\tHight Priority thread: {0}", i);
Thread.Sleep(500);
}
// I was curious to know if a process has more work load !
//chek it out, and
//Process newProc = Process.Start("iexplore.exe");
Process newProc = Process.Start("notepad.exe");
Console.WriteLine("\t\tNew process started...Must close the application
you open with");
newProc.WaitForExit();
newProc.Close();
}
}
|
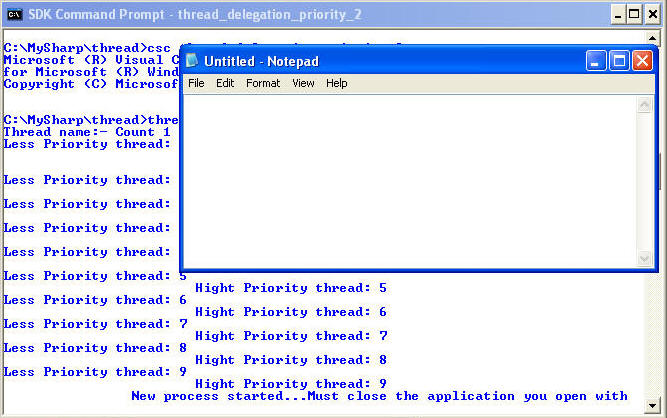 |
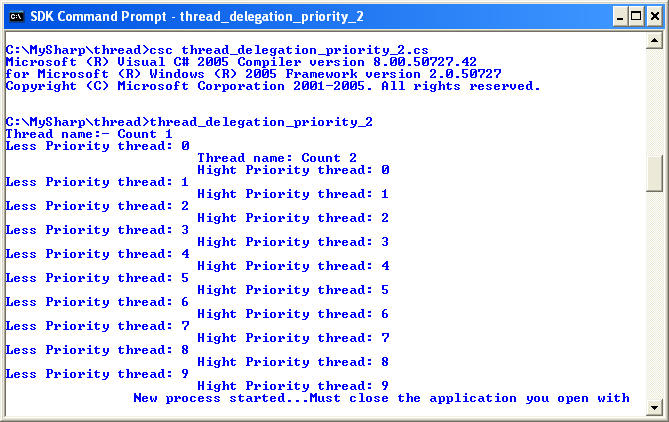 |
|
Runt with no sleep |
using System;
using System.Threading;
using System.Diagnostics;
// csc thread_delegation_priority_3.cs
//also imples to ThreadStart examples
public class Test
{
static void Main()
{
thread_count cycle = new thread_count();
///set up for count 1
ThreadStart job1 = new ThreadStart(cycle.Count1);
Thread thread1 = new Thread(job1);
thread1.Name ="Count 1 ";
//set up for count 2
ThreadStart job2 = new ThreadStart(cycle.Count2);
Thread thread2 = new Thread(job2);
thread2.Name ="Count 2 ";
//
thread2.Priority = ThreadPriority.Highest;
thread1.Priority = ThreadPriority.Lowest;
thread1.Start();
thread2.Start();
//
Console.ReadLine();
}
}
public class thread_count
{
public void Count1()
{
Console.WriteLine ("Thread name:- {0}",Thread.CurrentThread.Name.ToString());
for (int i=0; i < 10; i++)
{
Console.WriteLine ("Less Priority thread: {0}", i);
//allow other threads to work with sleep
//Thread.Sleep(500);
}
}
public void Count2()
{
Console.WriteLine ("\t\t\tThread name: {0}",Thread.CurrentThread.Name.ToString());
for (int i=0; i < 10; i++)
{
Console.WriteLine ("\t\t\tHight Priority thread: {0}", i);
//hread.Sleep(500);
}
// I was curious to know if a process has more work load !
//chek it out, and
//Process newProc = Process.Start("iexplore.exe");
Process newProc = Process.Start("notepad.exe");
Console.WriteLine("\t\tNew process started...Must close the application
you open with");
newProc.WaitForExit();
newProc.Close();
}
}
|
Since "Count_1" had a head start, system switches to the thread with
priority. How ever the application associated with the prioritized thread
opened later then less prioritized thread.
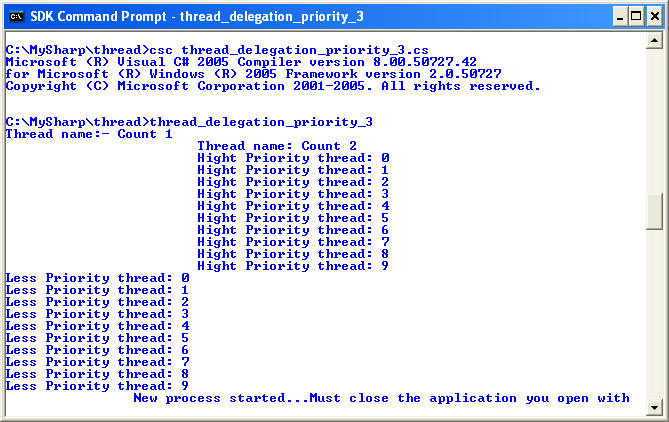
|
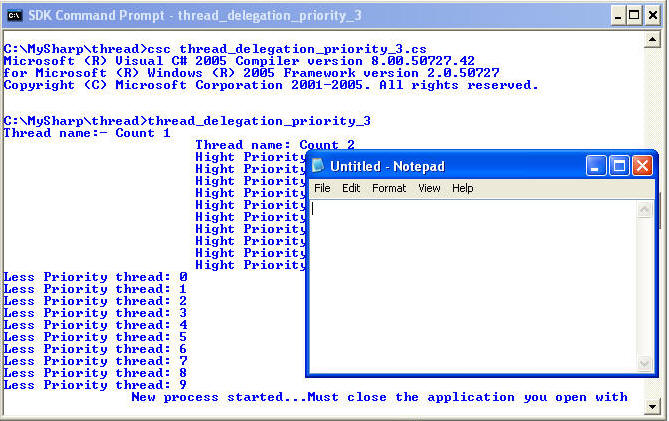 |
|