With ThreadStart class, you can avoid
explicit delegation to thread.
Note this
- delagate a job to a
- thread ThreadStart job = new ThreadStart(cycle.Count);
- Create a thread and link with delegation
- Thread thread = new Thread(job);
- You may name the thread (optional)
- thread.Name ="secondary thread ";
- Start the thread
|
MSDN Notes Beginning in version 2.0 of the
.NET Framework,
// it is
not necessary to create a delegate explicityly.
// Specify
the name of the method in the Thread constructor,
// and the
compiler selects the correct delegate. For example:
//
// Thread
newThread = new Thread(Work.DoWork);
|
|
The example below shows how to delegate a thread and schedules two
processes.
|
using System;
using System.Threading;
using System.Diagnostics;
// csc thread_delegation.cs
//also imples to ThreadStart examples
public class Test
{
static void Main()
{
thread_count cycle = new thread_count();
ThreadStart job = new ThreadStart(cycle.Count);
//job.Name= "Master counter" ;
//copy to anothr thread
Thread thread = new Thread(job);
thread.Name ="secondary thread ";
thread.Start();
for (int i=0; i < 3; i++)
{
Console.WriteLine ("Main thread:------- {0}", i);
Thread.Sleep(1000);
}
Console.ReadLine();
}
}
public class thread_count
{
public void Count()
{
Console.WriteLine ("name thread:---count down---- {0}",Thread.CurrentThread.Name.ToString());
for (int i=0; i < 10; i++)
{
Console.WriteLine ("Other thread: {0}", i);
Thread.Sleep(500);
}
//Process newProc = Process.Start("iexplore.exe");
Process newProc = Process.Start("notepad.exe");
Console.WriteLine("New process started...Must close the application you
open with");
newProc.WaitForExit();
newProc.Close();
}
}
|
The process continues as a countdown and then opens an application that
you must close. There are two system utilities are involved threading and
diagnostics. You can open any program and force the console windows remain
open till the applications are open.
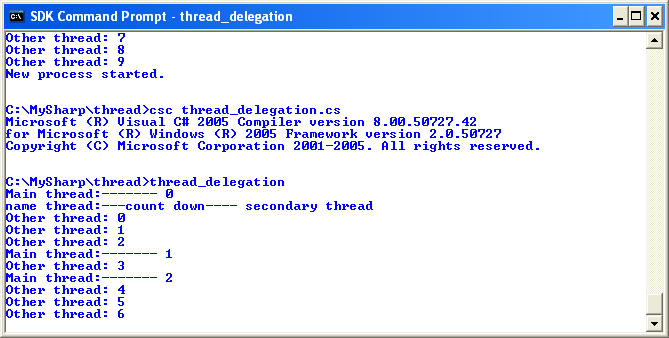
|
unless you close the application on the thread, cursor will not return
to console window. 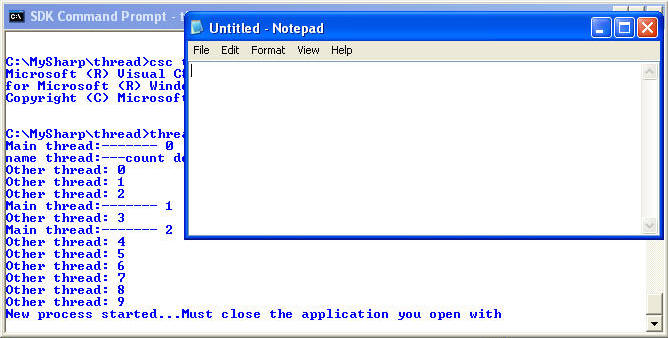
|
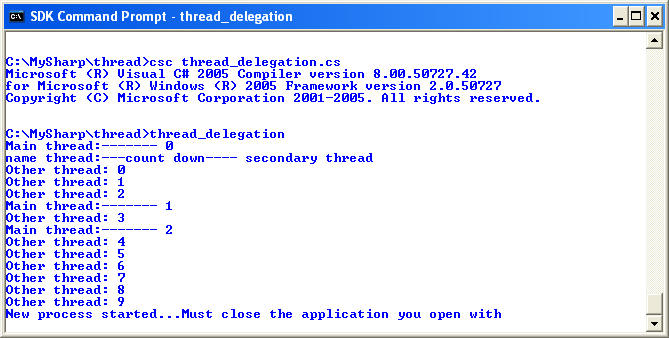 |
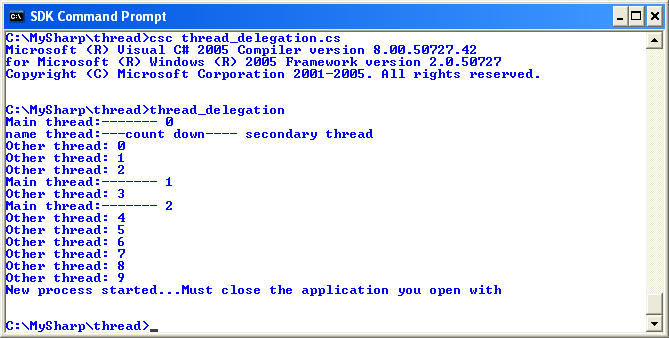 |
Below is the revised version of the same
using System;
using System.Threading;
using System.Diagnostics;
// csc thread_delegation_schedule.cs
//also imples to ThreadStart examples
public class Test
{
static void Main()
{
thread_count cycle = new thread_count();
ThreadStart job = new ThreadStart(cycle.Count);
//job.Name= "Master counter" ;
//copy to anothr thread
Thread thread = new Thread(job);
thread.Name ="secondary thread ";
thread.Start();
for (int i=0; i < 3; i++)
{
Console.Write("Thread no:{0}",Thread.CurrentThread.GetHashCode().ToString());
Console.WriteLine (" Main thread:--- {0}", i);
//pause intermitantly that allows another thread to work
//with in the life time of this thrad
Thread.Sleep(1000);
}
Console.ReadLine();
}
}
public class thread_count
{
public void Count()
{
Console.WriteLine ("name thread:---count down---- {0}",Thread.CurrentThread.Name.ToString());
for (int i=0; i < 10; i++)
{
Console.WriteLine ("Other thread: {0}", i);
Thread.Sleep(500);
}
//Process newProc = Process.Start("iexplore.exe");
Process newProc = Process.Start("notepad.exe");
Console.WriteLine("New process started...Must close the application you
open with");
newProc.WaitForExit();
newProc.Close();
}
}
|
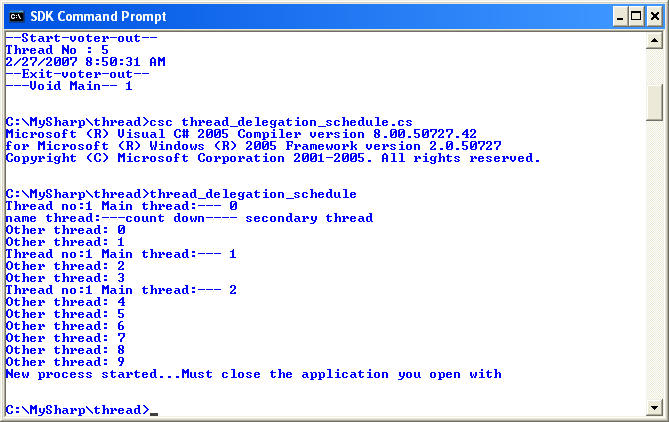 |
|
|