interface.htm |
- Interfaces is place or signature holder a group of related behaviors that can belong to any class or struct.
It is an effort to keep similar objects together.
- Example
interface team1
{
void MyFunction(string str);//this is a method
}
- Interfaces may contain methods, properties, events, indexers, or any combination of those four member types.
- An interface can not contain fields.
- The members of the Interfaces are public in default.
- Classes and structs can inherit from interfaces in a manner similar to how classes can inherit a base class or struct, with two exceptions:
- A class or struct can inherit more than one interface.
- When a class or struct inherits an interface, it inherits only the method names and signatures, because the interface itself contains no implementations. For example:
public class Minivan : Car,
IComparable
{
public
int CompareTo(object obj)
{
//implementation of CompareTo
return
0; //if the Minivans are equal
}
}
|
To implement an interface member,
|
//csc interface_1.cs
using System;
namespace soccer
{
interface team1
{
void MyFunction(string str);
}
interface team2
{
void MyFunction(string str);
}
class Test : team1,team2
{
public void MyFunction(string str)
{
Console.WriteLine("Hmm, I am not sure where to go : " + str);
}
}
class AppClass
{
public static void Main(string[] args)
{
string str = "player 1";
Test t=new Test();
team1 t1=(team1)t;
t1.MyFunction(str);
team2 t2=(team2)t;
t2.MyFunction(str);
}
}
} |
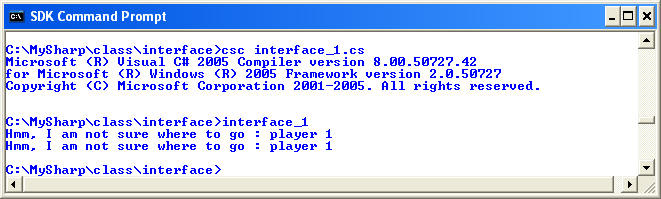 |
In the above scenario, we can use dot operator to point a method having similar name.
//csc interface_2.cs
using System;
namespace soccer
{
interface team1
{
void MyFunction(string str);
}
interface team2
{
void MyFunction(string str);
}
class Test : team1,team2
{
//can't use public void team1.MyFunction()
void team1.MyFunction(string str)
{
Console.WriteLine("Team 1 invites " + str);
}
void team2.MyFunction(string str)
{
Console.WriteLine("Team 2 invites " + str);
}
}
class AppClass
{
public static void Main(string[] args)
{
Console.WriteLine("Player for team 1 : ");
string s1 = Console.ReadLine();
Test t=new Test();
team1 t1=(team1)t;
Console.WriteLine("Player for team 2 : ");
string s2 = Console.ReadLine();
t1.MyFunction(s1);
team2 t2=(team2)t;
t2.MyFunction(s2);
}
}
}
|
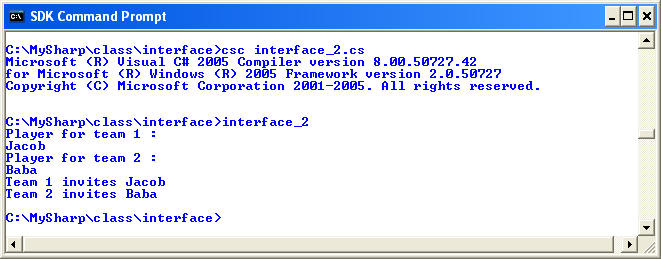 |
|