constructor_property.htm |
In this example we show the followings
- properties read only : get
- using constructor in properties
- note since we asked specific constructor, default will remain
silent
- public clsPro(double dVal) and SET properties compared
|
using System;
//csc property_1.cs
public class clsPro
{
private double gp = 0;
public clsPro() { Console.WriteLine("default constructor evoked");}
~clsPro() { Console.WriteLine("destructor evoked");}
public clsPro(double dVal)
{
gp = dVal;
Console.WriteLine("Through another constructor(dVal) evoked");
}
public double GP
{
get
{
Console.WriteLine("Passing through Property");
return gp;
}
}
}
class Test
{
static void Main(string[] args)
{
Console.Write("Read some double data : ");
string str = Console.ReadLine();
double dd = double.Parse(str);
clsPro pr= new clsPro(dd);
Console.WriteLine("Property Value Read only : "+ pr.GP);
}
}
|
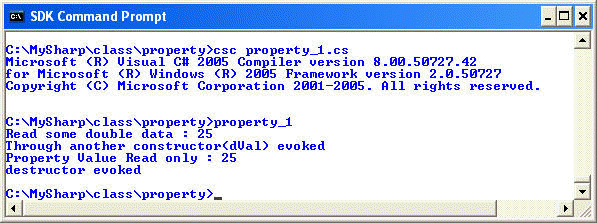 |
in this example we call two constructors we created
using System;
//csc property_1b.cs
public class clsPro
{
private double gp = 0;
public clsPro()
{ Console.WriteLine("default constructor evoked");}
~clsPro() { Console.WriteLine("destructor evoked");}
public clsPro(double dVal)
{
gp = dVal;
Console.WriteLine("Through another constructor(dVal) evoked");
}
public double GP
{
get
{
Console.WriteLine("Passing through Property");
return gp;
}
}
}
class Test
{
static void Main(string[] args)
{
Console.Write("Read some double data : ");
string str = Console.ReadLine();
double dd = double.Parse(str);
//summon default constructor
clsPro p= new clsPro();
clsPro pr= new clsPro(dd);
Console.WriteLine
("Property Value Read only : "+ pr.GP);
}
}
|
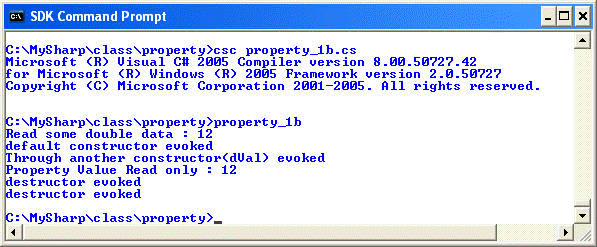 |
Set with |
using System;
//write only
//csc property_1c.cs
public class clsPro
{
private double sp = 0;
public clsPro()
{ Console.WriteLine("default constructor evoked");}
~clsPro()
{ Console.WriteLine("destructor evoked");}
public double SP
{
set
{
sp = value;
Console.WriteLine("Passing through Write Only : "+ sp);
}
}
}
class Test
{
public static void Main(string[] args)
{
Console.Write("Read some double data : ");
string str = Console.ReadLine();
double dd = double.Parse(str);
clsPro pr = new clsPro();
pr.SP = dd;
}
}
|
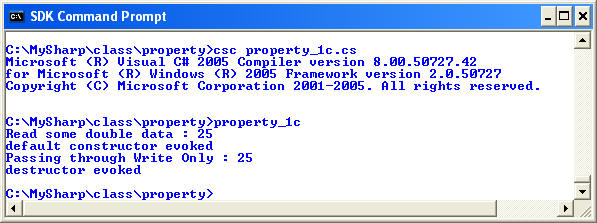 |