Below is the example of constructor over loading, you can call teh
constructor of your choice to manipulate some method or function in the
class. |
|
using System;
using System.Collections;
//csc constructor_overloading_1.cs
namespace Constructor_overloading
{
class test {
public static void Main()
{
string s ="Helo Wrold";
Simple sc = new Simple();
Console.WriteLine(sc.n2);
Simple sc1 = new Simple(1);
//Console.WriteLine(sc1.n2);
Simple sc3 = new Simple(1,2);
Console.WriteLine(sc3.n3);
Simple sc4 = new Simple(s);
Console.WriteLine(sc4.s2);
Console.ReadLine();
}
}
public class Simple {
public int n2, n3;
public string s2;
//this constructor should be public to be accessed
//behavior is not predictable
public Simple() { Console.Write("constructor--default--" ); n2 = 25; }
public Simple(int n1) { Console.WriteLine("constructor-int-overloading--"
+ (n3= 15));}
public Simple(int x, int y) {
Console.WriteLine("constructor-int-int-overloading--");n3= 15; }
public Simple(string s1) { Console.WriteLine("constructor-string-overloading--");
s1= "it is not a method will now show up";
this.s2 = s1;}
}
}
|
|
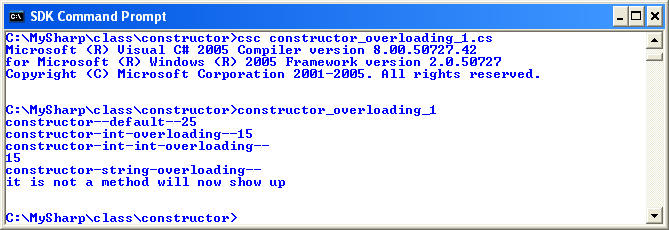 |
|
A |
|
Consider Second example
using System;
//csc constructor_opverloading_2.cs
namespace constructor_opverloading
{
class multiply
{
public int z;
private int x, y;
public multiply() { Console.WriteLine(" constructor original"); }
public multiply(int input1, int input2)
{
this.x = input1;
this.y = input2;
{ Console.WriteLine(" constructor overloaded ");}
}
public int method_1(int xx, int yy)
{
x = xx;
y = yy;
z = x * y;
return z;
}
}
class test
{
static void Main(string[] args)
{
//instantiating a class with two integers are parameter
// doing this compiler will look for custom/specific constructor
multiply mp1 = new multiply(1,2);
//multiply mp = new multiply(); has been
remarked
//mp1= mp;
Console.Write("enter first numbers ");
int n1, n2;
n1 = Int32.Parse(Console.ReadLine());
Console.Write("enter second number ");
n2 = Int32.Parse(Console.ReadLine());
Console.WriteLine("Total is : " +
mp1.method_1(n1, n2));
Console.ReadLine();
}
}
}
|
using System;
//csc constructor_opverloading_3.cs
namespace constructor_opverloading
{
class multiply
{
public int z;
private int x, y;
public multiply() { Console.WriteLine(" constructor original"); }
public multiply(int input1, int input2)
{
this.x = input1;
this.y = input2;
{ Console.WriteLine(" constructor overloaded ");}
}
public int method_1(int xx, int yy)
{
x = xx;
y = yy;
z = x * y;
return z;
}
}
class test
{
static void Main(string[] args)
{
//multiply mp1 = new multiply(1,2); has been
remarked
multiply mp = new multiply();
//mp1= mp;
Console.Write("enter first numbers ");
int n1, n2;
n1 = Int32.Parse(Console.ReadLine());
Console.Write("enter second number ");
n2 = Int32.Parse(Console.ReadLine());
Console.WriteLine("Total is : " + mp.method_1(n1, n2));
Console.ReadLine();
}
}
}
|
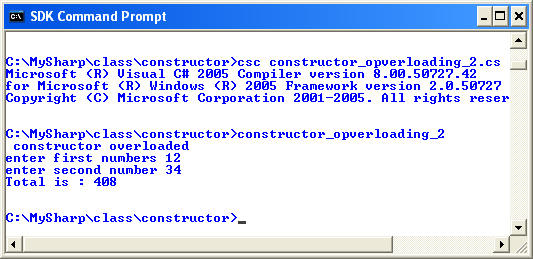 |
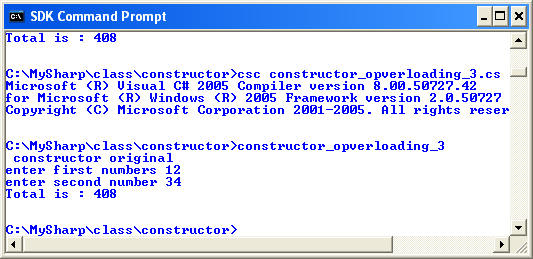 |
The example below has no purpose but to show some nature and simple
manipulation with class constructor
using System;
//csc constructor_opverloading_4.cs
namespace constructor_opverloading
{
class multiply
{
public int z;
private int x, y;
private string str;
public multiply() { Console.WriteLine(" constructor original"); }
public multiply(string s){ Console.WriteLine(" constructor string");}
public multiply(int input1, int input2)
{
this.x = input1;
this.y = input2;
{ Console.WriteLine(" constructor overloaded ");}
}
~multiply(){Console.WriteLine(" destructed "); }
public int method_1(int xx, int yy)
{
x = xx;
y = yy;
z = x * y;
Console.WriteLine("received string: " + str);
return z;
}
public int method_1(int xx)
{
int yy = 34;
x = xx;
y = yy;
z = x * y;
return z;
}
public string method_1(string s){ this.str = s; return str;
Console.WriteLine("what is y " + this.y); }
class test
{
static void Main(string[] args)
{
multiply mp1 = new multiply(1,2);
multiply mp = new multiply();
multiply mp2 = new multiply("new string");
mp= mp1=mp2;
Console.Write("enter first numbers ");
//expect worning while running command line tool
int n1, n2;
n1 = Int32.Parse(Console.ReadLine());
Console.Write("enter second number ");
n2 = Int32.Parse(Console.ReadLine());
Console.WriteLine("Noparam Total is : " + mp.method_1(n1));
Console.WriteLine("String sent out : " + mp.method_1("Hello World"));
Console.WriteLine("Param Total is : " + mp.method_1(n1, n2));
//string str =
//MessageBox.Show(" Total is " + mp.z);
Console.ReadLine();
}
}
}
}
|
|
Note all the constructors were called, both constructor and
methods overloaded. We have no control oon destructor, it will sweep/kill
constructors. As the integers variable were not instantiated command line
tool will throw a warning. |
|
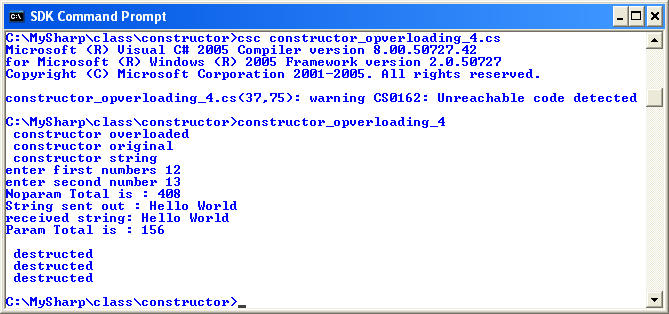 |
|