void_or_return.htm |
using System;
//void_or_return.cs
public class test_com_para
{
public static int Main(string[] str)
{
Console.WriteLine("You entered {0} command line arguments:", str.Length );
for (int i=0; i < str.Length; i++)
{
Console.WriteLine("-----------------");
Console.WriteLine("Argument {0}", i);
Console.WriteLine("-----------------");
Console.WriteLine("{0}", str[i]);
}
return 0;
}
}
|
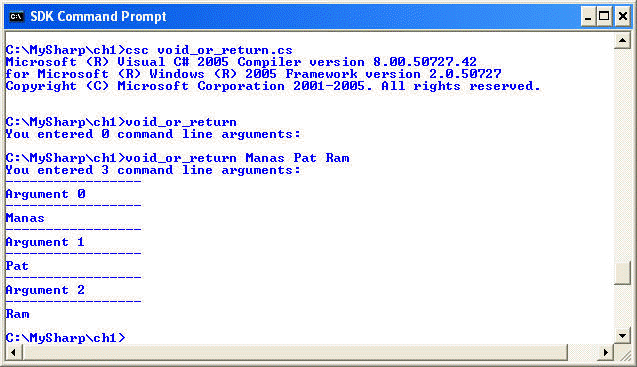 |
Let us consider the above code, and it would have been written like
this, red lines are updated or eidted |
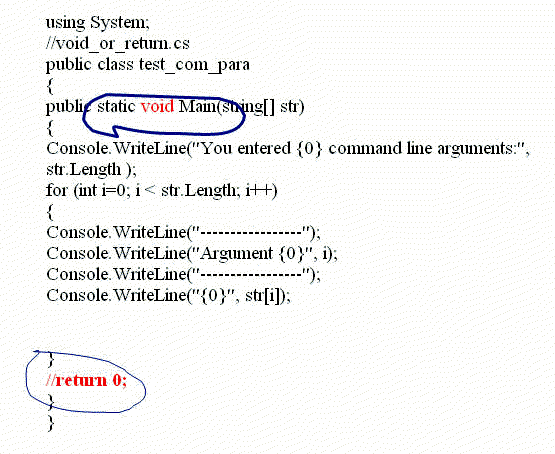 |
Let us edit, rename, save and run . using System;
//void_or_return_b.cs
public class test_com_para
{
public static void Main(string[] str)
{
Console.WriteLine("You entered {0} command line arguments:", str.Length );
for (int i=0; i < str.Length; i++)
{
Console.WriteLine("-----------------");
Console.WriteLine("Argument {0}", i);
Console.WriteLine("-----------------");
Console.WriteLine("{0}", str[i]);
}
//return 0;
}
}
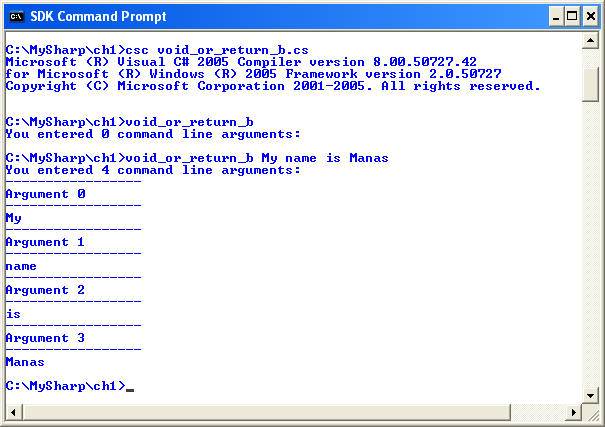
|
You may also code like this where the users does not enter a value, the
program will terminate wont show the error like this. using System;
//force_return.cs
public class force_return
{
public static int Main(string[] str)
{
if (str.Length == 0)
{
Console.WriteLine("Please enter a numeric argument.");
return 1;
}
Console.WriteLine("You entered {0} command line arguments:", str.Length );
for (int i=0; i < str.Length; i++)
{
Console.WriteLine("-----------------");
Console.WriteLine("Argument {0}", i);
Console.WriteLine("-----------------");
Console.WriteLine("{0}", str[i]);
}
return 0;
}
}
|
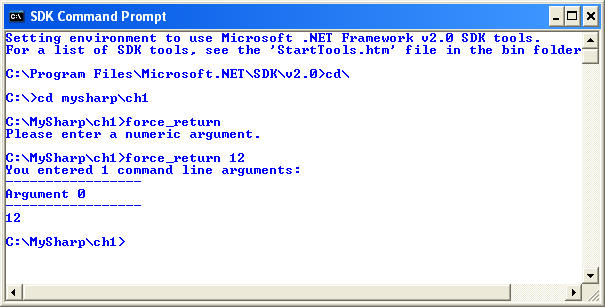 |
|
Consider this codes, where we are using two classes, one class calls
another and the other class does the job sends the results to the caller.
using System;
//csc void_or_return_c.cs
public class remote
{
public int m_add(int a, int b )
{
int f = (a + b );
return f;
}
public int m_mult(int a, int b )
{
int f = 0;
if ( a > b ){ f = a * b; }
return f;
}
}
/// end of the remote class
class test
{
static void Main()
{
Console.Write("Enter First Number : ");
int n1 = int.Parse(Console.ReadLine());
Console.Write("Enter First Number : ");
int n2 = int.Parse(Console.ReadLine());
// declare a variable of the class type data
remote r ;
//late biniding ! not exactly, but now object is created
r = new remote();
Console.WriteLine("This adds two numbers : " + r.m_add(n1,n2));
Console.WriteLine("This multiplies two numbers : " + r.m_mult(n1,n2));
}
}
|
In this example, the method returns a value to the calling function, and
caller prints at command line. For similar example view this example
Return String
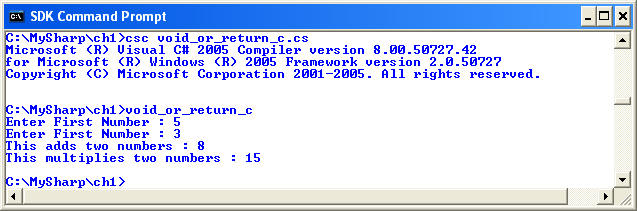 |
C# unlike VB counter part does not handle late binding with "Option
Strict Off directive", however, with Reflection namespace C# codes can
determine the type of member is running and allow late binding. |