Step: 3 Runtime Views:
a) Imposing size constraints: Testing an input which was more than 15 characters long. As
defined in the “if statement block”, the application was terminated
early because the string was longer than 15 characters.
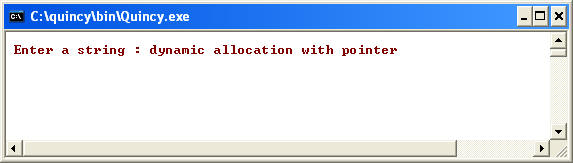
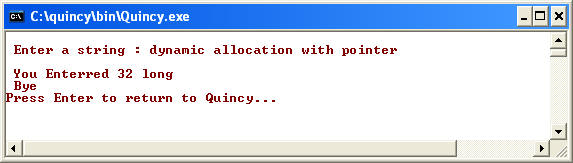
b) Passing string value to a pointer: Note that original
string “Hello World” did not mutate, when passed to a pointer with
new operator. It is apparent that one pointer passes the data to
another pointer, as a deep copy, and change in one pointer would be
reflected on the other.
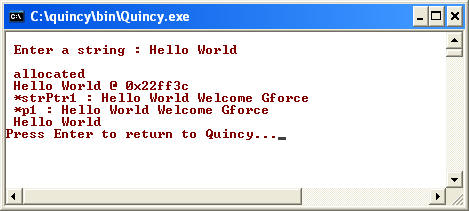
The illustration below, shows that the runtime reference of
"strPter1" allocated a new, location on heap, stored the value a
string object, and did not hold the reference of the string-object
"str1". Therefore, the value of the string-object "str1" remained
unaltered.
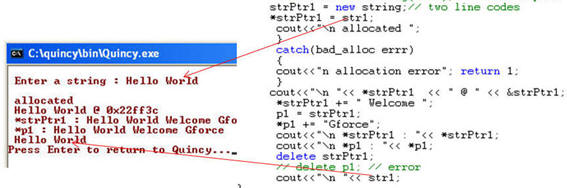
However, the data exchange between the two pointers occurred as a
deep-copy, and the reference of the object (pointer strPtr1) was
passed to the other (pointer p1). As
a result, any change in one of the two objects was reflected on
the other.