C++- pointer reference and dereference |
Objective
- pointier basics:
- pointer is classified under derived data type, along
with array and reference
- indirection pointer : *
- dereference pointer: (also know as structure
pointer operator)
-> ( member selection(pointer) ( example structure_object->member_variable = 10; )
This will set reference to the class . The same can be
acheived with DOT operator with (----) .
(structure_object)member_variable = 10;
- DOT operator
structure_object.member_variable = 10 ;
this directs to member_variable, since DOT precedes
INDIRECTION operator, the pointer will try to deference the
member_variable than the structure_object.
- indirection and address of operators : *&
- these pointers are unary type, meaning that these would
appear in front of the operand like *n1, &p1 or classobject->variable
= 10;
- pointer to integer data type
|
what is Pointer
A pointer is actually holds an address of variable in the computer memory.
- reference operator "&" also known as address of
operator.
- indirection operator "*" also known as "value pointed by"
: this allows to access a value through a pointer. (This is
different from multiplication * operator ) .
- deference operator -> (
myPointerToClass1.htm )
"from -CPP Essentials-->A pointer is simply the address of an object
in memory. Generally, objectscan be accessed in two ways: directly by
their symbolic name, or indirectly througha pointer. The act of getting
to an object via a pointer to it, is called dereferencingthe pointer.
Pointer variables are defined to point to objects of a specific type
sothat when the pointer is dereferenced, a typed object is obtained."
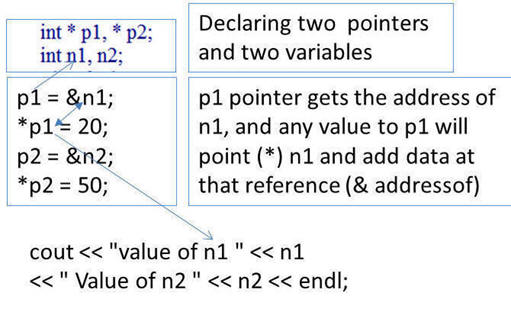
|
#include <iostream>
#include <stdio.h>
#include <fstream>
using namespace std;
//pointer_1.cpp
//size_t fread ( void * ptr, size_t size, size_t count, FILE * stream );
int main()
{
int * p1, * p2;
int n1, n2;
p1 = &n1;
*p1 = 20;
p2 = &n2;
*p2 = 50;
cout << "value of n1 " << n1 << " Value of n2 " << n2 <<endl;
cout << "address of n1 " << &n1 <<endl; cout<< "address of p1 " << p1;
return 0;
}
|
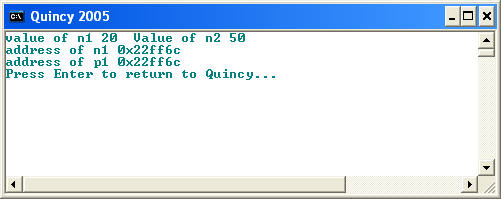 |
In the above example you note that p1 and n1 both are pointing to the
same address |