Step 4 Brief discussion: In one of
the previous example (gets_unsafe1.htm ), we noticed that the
function gets(param) which uses an array as a parameter, to store character
sequence (c-string) of undefined size, and can ignore the size
constraints of an array.
Using Pointers: A pointer is a powerful tool, but
should be used wisely, and should not be used as an open ended
variable, as shown in the image below.
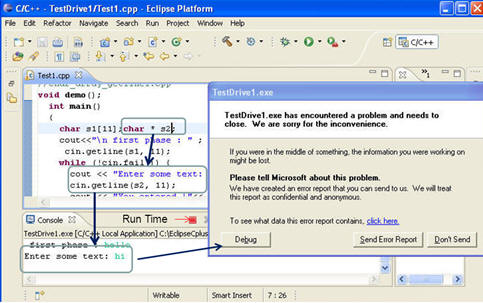
A pointer must be provided with the
address of the data allocated by the system.
char ch1[10]; char *ptr;
ptr = &ch1[0]; // pointing at the first element of the array*/
In this regard, the following
definitions for "ch1" are functionally similar.
char *ch1; ch1 = (char
*)malloc(100); OR char ch1[100];
char *ch1 and char ch[0] are syntactically different ways
of referring the same value.
In the previous example (
msize_realloc_malloc1.htm ) , we used a pointer with a
predefined size, "ch1 =
(char *)malloc(100);", then reallocated memories from the
heap, freeing unused memories back to the system.
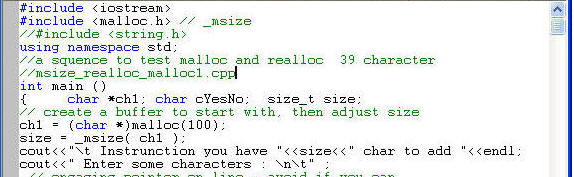
Function strcpy(...) : One The major advantage of
C++ I/O operation rests on the use of "=" operations, where a
soft-copy of one string can be passed over to another string.
string s1= " Some
value" ; string s2 ; char ch1[10]; char ch2[10]; char *p1, *p2;
s2= s1; // allowed, a soft copy is created.
ch1=ch2; // not allowed
ch1[10]=ch2[10]; // compiles but ch1[10] will remain empty
p1 = (char*) malloc(strlen(ch1)+1);
p2= p2; // allowed, creates a deep copy
strcpy(p1,ch1);//allowed, array to pointer.
The function strcpy(....,...), offers an easiest way to copy the
reference of one array to another array or a pointer. This
function copies the C-string data-source into the another array or
pointer, including the null character.
Restricting oversized string: In
the current example, an array of static size was reinforced with a
statement block. If the users exceed the
declared size, the application would terminate before allocating memories
on the heap.
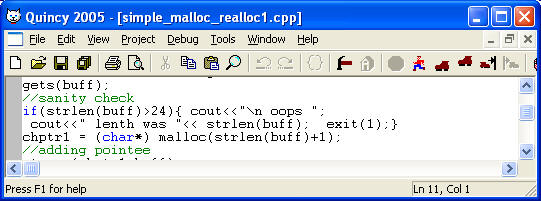
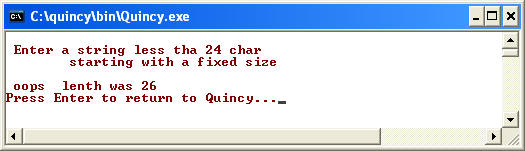
When users added an input which did not
exceed the size-limit, the input was allocated to a memory location,
so that an internal text, "Good Job", could be appended with the
help of reallocation function.