C++: About Header |
Consider the following codes
#include <iostream>
//first.cpp
int main()
{
std::cout << "Welcome to C++!\n";
return 0; // indicate that program ended successfully
}
|
Inclusion of Header Files (#include)
The line with #include notation, allows the programmer to call the
contents of one program within an another program. The C++ compiler
enforces that all the objects are to be declared before use. The the include directives set a
reference to the other program in the beginning of the code, and these
programs or routines are
processed before compiling the local code. Since, all these referred
programs in directives are
processed before the current code, these are known as Preprocessors or
Macros.
|
Header Files:
- Standard Template Library
- Why ? Just like your face, header files carries all
predefined conditions those are acceptable to the compiler. And
looking at your face that is attached to a name, your friend will know
who you are. This remain headers remain constant all along, but they
are not supposed to replace constant data type.
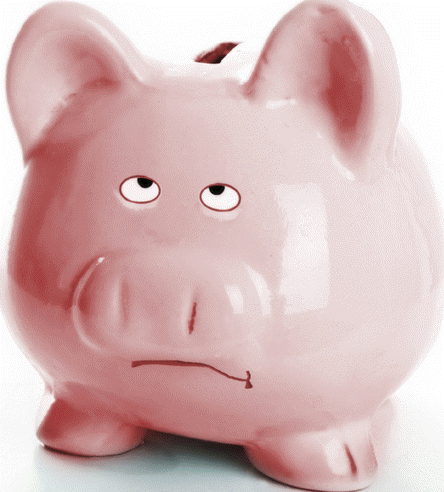
- Preprocessor or Macros
- More defined designations before compiling

- Meaning more elaborated and predefined conditions those should be
checked out before checking on the rest of the code in a
function.
|
Standard Template Library |
|
- ostream
- queue
- set
- sstream
|
- stack
- stdexcept
- streambuf
- string
|
- strstream
- typeinfo
- utility
- valarray
|
|
- algorithm
- bitset
- complex
- deque
|
- exception
- fstream
- functional
- iomanip
|
- ios
- iosfwd
- iostream
- istream
|
- iterator
- limits
- list
- locale
|
|
Preprocessors OR Macros: Preprocessor directives are used in the
headers, and are always
preceded by a pound sign (#). The preprocessors are executed before
the actual compilation of code initiates, some standard preprocessors are
listed below
|
Preprocessors OR Macros |
- #define
- #elif
- #else
- #endif
|
- #error
- #if
- #ifdef
- #ifndef
|
- #include
- #line
- #pragma
- #undef
|
|
C++ also includes the
Standard C Library: These libraries are also preceded by #include directives.
|
|
|
|
|
|
|
|
|