C++: Variables and Data types |
Objectives:Variable are used to hold the data that you would need to present to the
compiler to get your desired result
|
Consider this case
#include <iostream>
#include <string>
using namespace std;
//variable_1.cpp
int main()
{
int variable = 24;
char letter = 'A';
string str = "hello";
double d1 = 1.2;
float f1 = 1.2345678;
cout <<"hello world--from cout "<<
variable << "\t"<<letter << "\n"<< str <<endl;
cout <<" size of char " << sizeof(char) <<"\n";
cout <<" size of int " << sizeof(int) <<"\n";
cout <<" size of letter " << sizeof(letter) <<"\n";
cout <<" size of variable " << sizeof(variable) <<"\n";
return 0;
}
|
the results are shown below. 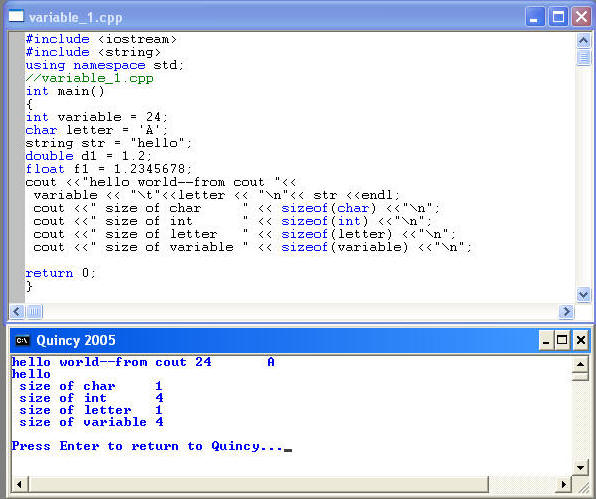
|
In the above example, if we see that we identify a data type whether it
is a numeral or alpha ( character or words), later in the routine we use
that identifier to display the value associated with it. |
Now let us consider other type of data type |
#include <iostream>
#include <string>
using namespace std;
//variable_2.cpp
int main()
{
int variable = 24;
char letter = 'A';
string str = "hello";
double d1 = 1.2;
float f1 = 1.2345678;
cout <<"hello world--from cout "<<
variable << "\t"<<letter << "\n"<< str <<endl;
cout <<" size of char " << sizeof(char) <<"\n";
cout <<" size of int " << sizeof(int) <<"\n";
cout <<" size of letter " << sizeof(letter) <<"\n";
cout <<" size of variable " << sizeof(variable) <<"\n";
cout <<" size of string " << sizeof(string) <<"\n";
cout <<" size of str " << sizeof(str) <<"\n";
cout <<" size of double " << sizeof(double) <<"\n";
cout <<" size of d1 " << sizeof(d1) <<"\n";
cout <<" size of float " << sizeof(float) <<"\n";
cout <<" size of f1 " << sizeof(f1) <<"\n";
return 0;
}
|
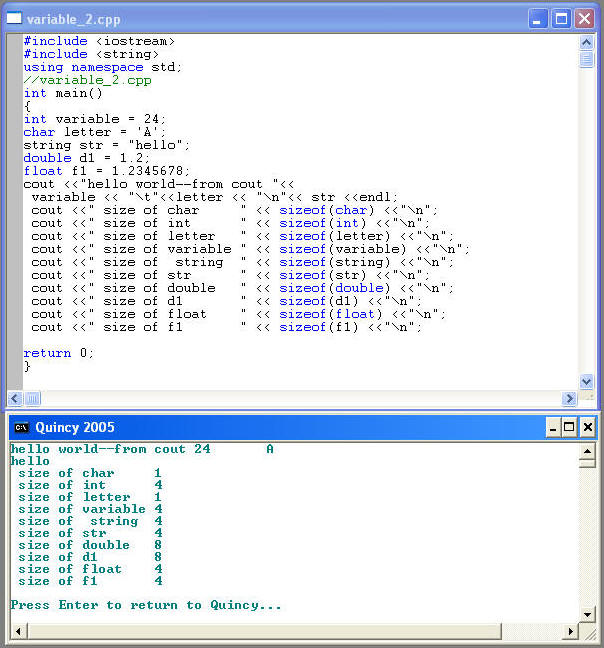 |
As we realize that we have data that would have some signature type like
int or char, the value assigned to these variables are saved in memory
location and you can view it too. Link |
Now know more about data types : Link |
|
|